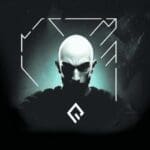
CSS Variables: How To Create Reusable CSS Values
Introduction
CSS variables, also known as custom properties, offer web developers a powerful and flexible way to create reusable CSS values. By using CSS variables, developers can establish consistent styles that are easy to maintain, modify, and scale. In this article, we'll discuss the basics of understanding CSS variables and how to create reusable values for color schemes, typography, layouts, and responsive designs.
Understanding CSS Variables
To use CSS variables effectively, it's important to understand their fundamentals. We'll discuss the syntax for defining and using variables, along with their scope and inheritance characteristics.
Defining CSS Variables
The syntax for defining CSS variables begins with two dashes (--
) as a prefix. Variables are typically declared within a selector, such as the :root
pseudo-class, which represents the document's root element.
Here's an example of declaring a CSS variable:
:root {
--primary-color: #f06;
}
In this example, we've created a variable called --primary-color
and assigned it the value #f06
.
Using CSS Variables
To use a CSS variable, the var()
function is employed. This function allows you to retrieve the value of a variable and apply it to a property.
Here's an example of using the --primary-color
variable we defined earlier:
button {
background-color: var(--primary-color);
}
In this example, we've set the background-color
property of the button
element to the value of our --primary-color
variable.
Scope and Inheritance
CSS variables are subject to the same scoping rules as other CSS properties. Variables declared within an element are only accessible to that element and its descendants. Additionally, CSS variables inherit their values from parent elements.
For example, consider the following HTML and CSS:
<div class="container">
<div class="box"></div>
</div>
.container {
--color: red;
}
.box {
background-color: var(--color);
}
In this case, the --color
variable is declared within the .container
class. The .box
class, being a descendant of .container
, can access and use the value of the --color
variable. Consequently, the background color of the .box
element will be set to red
.
Creating Reusable CSS Values
Now that we understand the basics of defining and using CSS variables, let's explore how to create reusable values for various aspects of a design system, such as color schemes, typography, layouts, and responsive designs.
Color Schemes
CSS variables make it easy to create and maintain color schemes for your projects. You can define a set of colors in your stylesheet and then apply these colors consistently throughout your project.
Here's an example of creating a color scheme with CSS variables:
:root {
--primary-color: #2c3e50;
--secondary-color: #3498db;
--background-color: #ecf0f1;
--text-color: #333;
--accent-color: #f1c40f;
}
Now that we've defined our color scheme, we can apply these variables to elements and components in our project:
header {
background-color: var(--primary-color);
}
nav a {
color: var(--secondary-color);
}
body {
background-color: var(--background-color);
color: var(--text-color);
}
button {
background-color: var(--accent-color);
color: var(--text-color);
}
Typography
Typography is another crucial aspect of a design system. CSS variables can be used to create and manage reusable values for font sizes, font families, and line heights, among other properties.
Here's an example of using CSS variables to define typography:
:root {
--font-family-body: 'Helvetica Neue', Helvetica, Arial, sans-serif;
--font-family-heading: 'Garamond', 'Times New Roman', serif;
--font-size-base: 16px;
--font-size-h1: 2rem;
--font-size-h2: 1.5rem;
--line-height: 1.5;
}
With these variables in place, we can apply our typography styles to appropriate elements and components:
body {
font-family: var(--font-family-body);
font-size: var(--font-size-base);
line-height: var(--line-height);
}
h1 {
font-family: var(--font-family-heading);
font-size: var(--font-size-h1);
}
h2 {
font-family: var(--font-family-heading);
font-size: var(--font-size-h2);
}
Layout and Spacing
When creating reusable CSS values, managing layout and spacing properties is essential for maintaining consistent designs. CSS variables can be used to control grid gaps, margins, paddings, and other layout-related properties.
Here's an example of defining layout and spacing variables:
:root {
--grid-gap: 16px;
--margin-small: 8px;
--margin-large: 32px;
--padding-small: 8px;
--padding-medium: 16px;
--padding-large: 24px;
}
With these variables, we can apply consistent layout and spacing values throughout our design system:
.container {
display: grid;
grid-gap: var(--grid-gap);
}
.card {
margin: var(--margin-small) var(--margin-large);
padding: var(--padding-medium);
}
.modal {
padding: var(--padding-large);
}
Responsive Design
CSS variables can also be employed to create responsive designs by adjusting their values using media queries. This offers a powerful and flexible way to control styles based on viewport size or other conditions.
Here's an example of how to adjust CSS variable values for different screen widths:
:root {
--font-size-base: 16px;
}
@media (min-width: 768px) {
:root {
--font-size-base: 18px;
}
}
@media (min-width: 1024px) {
:root {
--font-size-base: 20px;
}
}
In this example, we're setting the base font size to 16px on small screens, and increasing the font size to 18px for screens with a minimum width of 768px, and 20px for screens with a minimum width of 1024px. By adjusting the value of the --font-size-base
variable, we can create a responsive design using a single variable throughout our project.
Best Practices and Tips
Using CSS variables effectively involves adhering to best practices and tips that improve maintainability and flexibility. In this section, we'll discuss naming conventions, organizing and structuring variables, using fallback values, and ensuring compatibility with older browsers.
Naming Conventions
Adopting consistent naming conventions for CSS variables enhances readability and maintainability. A well-structured naming strategy makes it easier to identify the purpose of a variable and its relation to other variables.
Some popular naming conventions include:
- Prefixing variables with category or type: E.g.,
-color-primary
,-font-size-base
,-spacing-small
. - Using hyphen-separated names for compound terms: E.g.,
-border-radius-large
,-header-height
. - Lowercase for basic variables and camelCase for complex ones: E.g.,
-primarycolor
,-fontSizeBase
(not as common as hyphen-separated names).
Choose a naming convention that suits your project and team, and stick to it for consistency.
Organizing and Structuring Variables
Group related CSS variables in a stylesheet to make them easier to manage and locate. You can also use comments to document variable usage and purpose, improving the readability of your code.
For example:
/* Color Variables */
:root {
--primary-color: #2c3e50;
--secondary-color: #3498db;
/* ...
}
/* Typography Variables */
:root {
--font-family-body: 'Helvetica Neue', Helvetica, Arial, sans-serif;
--font-family-heading: 'Garamond', 'Times New Roman', serif;
/* ...
}
/* Layout Variables */
:root {
--grid-gap: 16px;
--margin-small: 8px;
/* ...
}
Fallback Values
CSS variables may be undefined or assigned an invalid value. By utilizing the var()
function, you can provide a fallback value to handle such situations. A fallback value ensures that your designs display as intended, even if a variable value is missing or incorrect.
Here's the syntax for providing a fallback value:
property: var(--variable, fallback-value);
For example:
background-color: var(--boxed-layout-background, #fff);
In this case, if --boxed-layout-background
is undefined or has an invalid value, the background color will default to #fff
.
Compatibility and Fallbacks
Modern browsers mostly support CSS variables. However, older browsers, such as Internet Explorer, may not. Providing fallback styles for older browsers is essential for ensuring accessibility and a consistent user experience.
One method for providing fallback styles is to declare the property with its value before referencing the CSS variable:
.button {
background-color: #f06;
background-color: var(--primary-color, #f06);
}
In this example, browsers that don't support CSS variables will use the first background-color
value of #f06
. Browsers that support CSS variables will overwrite this value with the --primary-color
variable's value or the provided fallback value.
Conclusion
Creating reusable CSS values using CSS variables enhances the maintainability and scalability of your stylesheets by allowing you to establish consistent design systems. In this article, we covered the basics of understanding CSS variables, as well as creating reusable values for color schemes, typography, layouts, and responsive designs. We also touched on best practices for naming conventions, organizing and structuring variables, and providing fallback values for improved compatibility and user experience.
By leveraging CSS variables in your projects, you can create more efficient, maintainable, and scalable styles that improve the performance of your websites and applications.
Frequently Asked Questions
What are the benefits of using CSS variables?
CSS variables enable you to create reusable values for your stylesheets, promoting consistency, maintainability, and scalability. By adopting CSS variables, you can easily update and manage styles throughout your project, simplify responsive designs, and build modular design systems. Moreover, using CSS variables can improve code readability and organization.
Can I use CSS variables with JavaScript?
Yes, you can interact with CSS variables using JavaScript. With JavaScript, you can set, retrieve, and modify the values of CSS variables. To access a CSS variable through JavaScript, use the getPropertyValue()
and setProperty()
methods on the style
object of an element or in the CSSStyleDeclaration
interface.
// Get the value of a CSS variable
const rootStyles = getComputedStyle(document.documentElement);
const primaryColor = rootStyles.getPropertyValue('--primary-color').trim();
// Set the value of a CSS variable
document.documentElement.style.setProperty('--primary-color', '#f06');
Are there any performance implications of using CSS variables?
CSS variables are generally considered performant since they are a native browser feature. They eliminate the need for CSS preprocessors, reducing build time and the frontend toolchain complexity. Any potential performance hit is negligible compared to the maintainability, readability, and flexibility benefits CSS variables provide.
How do CSS variables differ from variables in CSS preprocessors like Sass or Less?
CSS variables are native to browsers and can be used without relying on a preprocessor like Sass or Less. Moreover, CSS variables provide dynamic capabilities during runtime and can be modified via JavaScript, enabling enhanced interactivity and responsiveness. In contrast, variables in CSS preprocessors like Sass or Less are static, meaning their values are compiled and cannot be changed after the initial compilation.
How do I structure my CSS variables for a large-scale project?
In large-scale projects, it's essential to organize and structure your variables thoughtfully. Here are a few recommendations:
- Follow consistent naming conventions to make variable usage clear and maintainable.
- Group related variables together and use comments to document their purpose and usage.
- Consider creating separate files for different variable categories like colors, typography, layout, breakpoints, etc., and import these files into your main stylesheet.
- Keep global variables in shared files, while component-specific variables can be placed in component-specific stylesheets.