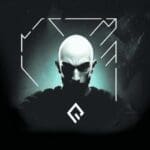
DOM Manipulation - How to Select, Create, Add, and Modify Elements
Introduction
Brief explanation of the DOM
The Document Object Model (DOM) is a programming interface that represents the structure of a web document or webpage. It allows web developers to interact with and manipulate web pages through a series of tree structures that represent the document's elements, attributes, and content. With DOM manipulation, developers can dynamically update web documents without the need for a page refresh. It enables the creation of interactive web applications, giving users a smoother and more seamless experience.
Importance of DOM manipulation in web development
DOM manipulation is a crucial skill for web developers, as it allows them to interact with web pages programmatically. This means developers can create and modify the HTML, CSS, and JavaScript code that makes up a web page, without having to edit the actual files directly. DOM manipulation provides the foundation for a variety of web development tasks, such as creating dynamic content, implementing user interactions, and generating new page elements based on user input.
Selecting DOM Elements
Before you can manipulate (change) a DOM element, you must first select it. DOM elements can be selected using various JavaScript methods, like getElementById
, getElementsByClassName
, querySelector
, and querySelectorAll
. Each of these methods has specific use cases and can help you target specific elements in the DOM.
Using document.getElementById, document.getElementsByClassName, document.querySelector, and document.querySelectorAll
document.getElementById
: This method is used to select an element with a specified ID. The ID should be unique within the document. It returns only the first matching element ornull
if no element with the given ID is found.
const headerElement = document.getElementById('header');
document.getElementsByClassName
: This method is used to select elements with a specified class. It returns an HTMLCollection (an array-like object) of all matching elements.
const listItemElements = document.getElementsByClassName('list-item');
document.querySelector
: This method is used to select the first element that matches a specified CSS selector (e.g., class, ID, or tag). If no matching elements are found, it returnsnull
.
const firstButtonElement = document.querySelector('.button');
document.querySelectorAll
: This method is similar toquerySelector
, but it returns a NodeList containing all matching elements rather than just the first match.
const allButtonElements = document.querySelectorAll('.button');
Practical examples of selecting elements
Here are a few practical examples of selecting DOM elements using the previously discussed methods:
// Select an element by its ID
const mainContainer = document.getElementById('mainContainer');
// Select all elements with a specific class
const cardElements = document.getElementsByClassName('card');
// Select the first element with a specific data attribute
const firstSliderElement = document.querySelector('[data-slider="1"]');
// Select all elements with a specific tag name
const paragraphElements = document.querySelectorAll('p');
In the next section, we will discuss how to create new DOM elements after selecting them.
Creating DOM Elements
To create interactive web applications, developers often need to create new DOM elements that are not present in the original HTML document. We can use JavaScript to create such elements, which can then be added to the DOM as required.
Using document.createElement, and setting properties
The document.createElement()
method is used to create new DOM elements. It takes one argument, the tag name of the element you want to create, and returns the newly created element.
Once the element is created, you can set its attributes, content, and other properties using various DOM manipulation methods.
// Create a new 'div' element
const newDiv = document.createElement('div');
// Set the class attribute for the newly created 'div' element
newDiv.setAttribute('class', 'container');
// Set the text content of the newly created 'div' element
newDiv.textContent = 'Hello, World!';
Practical examples of creating elements
Here are a few examples of creating new DOM elements and setting their properties:
// Create a new 'p' element and set its content
const paragraphElement = document.createElement('p');
paragraphElement.textContent = 'This is a new paragraph element.';
// Create a new 'img' element and set an image source attribute
const imageElement = document.createElement('img');
imageElement.setAttribute('src', '<https://example.com/image.jpg>');
imageElement.setAttribute('alt', 'Example Image');
// Create a new 'button' element and set its type, text content, and click event handler
const buttonElement = document.createElement('button');
buttonElement.setAttribute('type', 'button');
buttonElement.textContent = 'Click Me!';
buttonElement.addEventListener('click', () => {
alert('Button clicked!');
});
In the next section, we will discuss how to add these newly created DOM elements to a web page.
Adding DOM Elements
After creating new DOM elements, we need to add them to the DOM to make them visible on the web page. Developers can use methods like parentNode.appendChild()
and parentNode.insertBefore()
to achieve this.
Using parentNode.appendChild, parentNode.insertBefore
parentNode.appendChild()
: This method appends a new child element to the end of a specified parent element. It takes the new element as an argument and returns the appended child element.
const containerElement = document.getElementById('container');
const newElement = document.createElement('div');
newElement.textContent = 'I am a new div element!';
containerElement.appendChild(newElement);
parentNode.insertBefore()
: This method inserts a new child element before an existing child element within the specified parent element. It takes two arguments: the new element to be inserted and the existing child element before which the new element will be inserted. It returns the inserted child element.
const containerElement = document.getElementById('container');
const newElement = document.createElement('div');
newElement.textContent = 'I am a new div element!';
const existingElement = document.getElementById('referenceElement');
containerElement.insertBefore(newElement, existingElement);
Practical examples of adding elements
Here are a few examples of adding new DOM elements to a web page:
// Add a new paragraph element to the end of a 'div'
const containerElement = document.getElementById('container');
const paragraphElement = document.createElement('p');
paragraphElement.textContent = 'I am a new paragraph element.';
containerElement.appendChild(paragraphElement);
// Insert a new heading element before an existing paragraph element
const headingElement = document.createElement('h2');
headingElement.textContent = 'New Subheading';
const referenceElement = document.getElementById('referenceParagraph');
containerElement.insertBefore(headingElement, referenceElement);
In the next section, we will discuss how to modify existing DOM elements once they have been added to the page.
Modifying DOM Elements
After selecting and adding DOM elements to a web page, developers often need to modify them to achieve desired functionality or appearance. There are several methods for modifying DOM elements, including changing content, attributes, or styles.
Changing content with innerHTML, textContent
innerHTML
: TheinnerHTML
property allows you to set or get the HTML content of an element. This includes all the nested HTML tags and text content within the element.
// Add a new paragraph element using innerHTML
const containerElement = document.getElementById('container');
containerElement.innerHTML += '<p>I am a new paragraph element added using innerHTML.</p>';
// Change the content of an existing paragraph element using innerHTML
const paragraphElement = document.getElementById('existingParagraph');
paragraphElement.innerHTML = '<strong>I am a modified paragraph element.</strong>';
textContent
: ThetextContent
property allows you to set or get the text content of an element, without any HTML tags. It returns only the text inside the element and its descendants, excluding tags.
// Change the text content of an existing paragraph element using textContent
const paragraphElement = document.getElementById('existingParagraph');
paragraphElement.textContent = 'I am a modified paragraph element.';
Modifying attributes with setAttribute, removeAttribute
setAttribute
: ThesetAttribute()
method is used to add or modify an attribute on an element. It takes two arguments: the attribute name and the new value to be set.
const imageElement = document.getElementById('exampleImage');
imageElement.setAttribute('src', '<https://example.com/new-image.jpg>');
imageElement.setAttribute('alt', 'New example image');
removeAttribute
: TheremoveAttribute()
method is used to remove an attribute from an element. It takes one argument: the attribute name to be removed.
const containerElement = document.getElementById('container');
containerElement.removeAttribute('data-custom-attribute');
Practical examples of modifying elements
Here are a few examples of modifying DOM elements:
// Modify button text using textContent
const buttonTextElement = document.getElementById('buttonText');
buttonTextElement.textContent = 'New Button Text';
// Change the background color of an element using style
const containerElement = document.getElementById('container');
containerElement.style.backgroundColor = 'lightblue';
// Add a data attribute to an existing element using setAttribute
const customElement = document.getElementById('customElement');
customElement.setAttribute('data-custom-attribute', 'example value');
// Remove a class from an existing element
const containerElement = document.getElementById('container');
containerElement.classList.remove('oldClassName');
Practical Application: Building a Simple Web Page
Using the techniques covered in the previous sections, let's build a simple web page step-by-step, applying the following DOM manipulation tasks:
- Selecting elements
- Creating new elements
- Adding elements to the web page
- Modifying elements
- Selecting elements: First, we need to select the main container element in which we will add new elements.
const mainContainer = document.getElementById('mainContainer');
- Creating new elements: Next, we will create a new heading and paragraph element.
const headingElement = document.createElement('h1');
const paragraphElement = document.createElement('p');
- Adding elements to the web page: Now, we will add the newly created heading and paragraph elements to the main container element.
mainContainer.appendChild(headingElement);
mainContainer.appendChild(paragraphElement);
- Modifying elements: Finally, we will set the text content of the heading and paragraph elements.
headingElement.textContent = 'Welcome to our web page!';
paragraphElement.textContent = 'This is a simple web page created using DOM manipulation techniques.';
With these steps, we have built a simple web page using DOM manipulation, which demonstrates the power and flexibility of these techniques in creating dynamic web pages.
In the next section, we will discuss best practices for DOM manipulation and the importance of performance considerations.
Best Practices for DOM Manipulation
Manipulating the DOM is an essential part of web development, but it is crucial to follow best practices to ensure efficient and well-structured applications. The following general rules and guidelines will help you improve your DOM manipulation skills.
General rules and best practices
- Cache DOM references: Frequently accessing the DOM can have a detrimental impact on performance. To minimize this, store references to DOM elements in variables when they are used multiple times. By doing so, you reduce the number of times the DOM needs to be accessed.
// Instead of accessing the DOM repeatedly
document.getElementById('container').style.backgroundColor = 'red';
document.getElementById('container').style.padding = '1rem';
// Cache the DOM reference in a variable
const containerElement = document.getElementById('container');
containerElement.style.backgroundColor = 'red';
containerElement.style.padding = '1rem';
- Use the most specific selector: Selecting elements is an essential aspect of DOM manipulation, but the choice of selectors can impact performance. Always try to use the most specific selector (e.g.,
getElementById
orgetElementsByClassName
) to optimize performance. - Minimize DOM changes: Modifying the DOM's structure can cause the browser to recalculate layout, repaint the screen, and perform other tasks that may slow down the application. Minimize the number of changes to the DOM, and group these manipulations whenever possible.
- Use DocumentFragment for multiple insertions: When adding multiple elements to the DOM, use a
DocumentFragment
instance to minimize the browser's layout recalculations. ADocumentFragment
is a lightweight, container-less version of a document that can hold and manipulate multiple nodes without the overhead of a full-fledged document.
const fragment = document.createDocumentFragment();
const newElement1 = document.createElement('div');
const newElement2 = document.createElement('div');
fragment.appendChild(newElement1);
fragment.appendChild(newElement2);
document.getElementById('container').appendChild(fragment);
- Avoid using innerHTML for text content modifications: The
innerHTML
property has its uses, but it can be slow and inefficient when dealing with plain text content. Instead, use thetextContent
property for simple text updates.
Importance of efficiency and performance considerations
Efficient DOM manipulation is critical for maintaining a smooth user experience. Ensuring a responsive, performant website is an essential part of web development, and proper DOM manipulation techniques play a significant role in achieving this goal.
As developers, we must consider and optimize our applications to meet users' increasing expectations for fast-loading, responsive web pages. By understanding DOM manipulation and applying these best practices, you'll ensure a solid foundation upon which to build high-quality, interactive web applications.
Conclusion
DOM manipulation is a key skill for web developers, allowing you to create dynamic, interactive web applications and pages. This article explained how to select, create, add, and modify DOM elements using various techniques and methods.
As you progress as a web developer, it's essential to continue practicing and refining your DOM manipulation skills. Becoming proficient in this area will open up countless possibilities in building rich and interactive web experiences for users.
Keep learning, experimenting, and applying the best practices discussed in this article to enhance both your development skills and the performance of your web applications.
Frequently Asked Questions
Here are some commonly asked questions about DOM manipulation and their answers:
1. What are the differences between innerHTML, innerText, and textContent?
innerHTML
retrieves or sets the HTML content (including tags) of an element. innerText
retrieves or sets the visible text content of an element, considering CSS styles that affect the element's visibility. textContent
retrieves or sets the entire text content of an element and its descendants, without considering CSS styles.
2. How can I add an event listener to a DOM element?
Use the addEventListener
method to attach event listeners to DOM elements. The method takes two arguments: the event type (e.g., 'click', 'mouseover') and the and event handling function.
const buttonElement = document.getElementById('exampleButton');
buttonElement.addEventListener('click', () => {
alert('Button clicked!');
});
3. Can I manipulate the DOM using JavaScript libraries like jQuery?
Yes. Libraries like jQuery provide a more developer-friendly API for working with the DOM. However, it is essential to understand the basic DOM manipulation techniques using vanilla JavaScript, as it builds a strong foundation for using libraries and frameworks effectively.
4. How do I remove elements from the DOM?
You can remove a DOM element using the removeChild()
or remove()
method. The removeChild()
method is called on the parent node and takes the child node to be removed as an argument. The remove()
method is called directly on the element to be removed.
// Using removeChild
const parentElement = document.getElementById('parent');
const childElement = document.getElementById('childToRemove');
parentElement.removeChild(childElement);
// Using remove
const element = document.getElementById('elementToRemove');
element.remove();
5. How can I traverse the DOM?
Traversing the DOM means moving from one element to another element in the DOM tree structure. You can use properties like parentNode
, firstElementChild
, lastElementChild
, nextElementSibling
, and previousElementSibling
to traverse the DOM.
For example, to access the parent node of an element:
const childElement = document.getElementById('exampleChild');
const parentElement = childElement.parentNode;