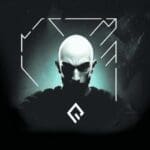
XMLHttpRequest Techniques Every JavaScript Developer Should Know
Introduction
XMLHttpRequest is a crucial part of web application development, as it enables developers to communicate with servers and perform actions such as fetching data or submitting forms. Mastering XMLHttpRequest techniques is essential for JavaScript developers to create efficient and responsive web applications. This article will provide an in-depth look at XMLHttpRequest, its key components, and several essential techniques that every JavaScript developer should know.
Understanding XMLHttpRequest
What is XMLHttpRequest?
XMLHttpRequest is a JavaScript object that allows developers to send HTTP requests and receive HTTP responses from servers within web applications. This enables web applications to perform tasks such as fetching data, submitting forms, and updating content without requiring a full page reload. XMLHttpRequest is the foundation of AJAX (Asynchronous JavaScript and XML), which powers many modern web applications.
Key Components of XMLHttpRequest
An XMLHttpRequest consists of several key components that enable communication with servers:
- XMLHttpRequest object: The main object that developers instantiate and configure to send requests and receive responses.
- Request methods: These are the HTTP methods used to perform actions on the server, such as GET, POST, PUT, DELETE, etc.
- Request URLs: The URL of the server endpoint that the request is sent to.
- Request headers: Additional metadata sent along with the request, such as content type and authentication tokens.
- Request and response data: The data sent to the server in the request payload and the data received from the server in the response.
Techniques Every JavaScript Developer Should Know
1. Creating and Configuring XMLHttpRequest
To use XMLHttpRequest, developers must first create an instance of the object and set up its configuration:
const xhr = new XMLHttpRequest();
xhr.open('GET', '<https://api.example.com/data>');
xhr.onreadystatechange = function () {
if (xhr.readyState === 4 && xhr.status === 200) {
console.log(JSON.parse(xhr.responseText));
}
};
xhr.send();
In this example, an XMLHttpRequest object is instantiated, the request method is set to 'GET', and the request URL is set to 'https://api.example.com/data'. The onreadystatechange
event listener is set up to handle the request completion, and the send()
method is called to send the request.
2. Sending GET Requests
GET requests are used to fetch data from the server. To send a GET request, developers must configure the XMLHttpRequest object with the GET method and handle the response data:
xhr.open('GET', '<https://api.example.com/data?query=value>');
In this example, the request URL includes query parameters. Developers should ensure that query parameters are properly URL-encoded to avoid issues with special characters.
3. Sending POST Requests
POST requests are used to send data to the server. To send a POST request, developers must configure the XMLHttpRequest object with the POST method, set the appropriate request headers, and handle the request payload:
xhr.open('POST', '<https://api.example.com/data>');
xhr.setRequestHeader('Content-Type', 'application/json;charset=UTF-8');
xhr.send(JSON.stringify({ key: 'value' }));
In this example, the request header is set to 'application/json', indicating that the request payload is in JSON format.
4. Handling Errors and Timeouts
Proper error handling is essential for a smooth user experience. Developers should configure timeout settings for XMLHttpRequest, handle network errors, and gracefully handle HTTP status errors:
xhr.timeout = 5000; // Set the timeout to 5 seconds
xhr.ontimeout = function () {
console.error('Request timed out');
};
xhr.onerror = function () {
console.error('Network error');
};
xhr.onreadystatechange = function () {
if (xhr.readyState === 4) {
if (xhr.status >= 200 && xhr.status < 300) {
console.log(JSON.parse(xhr.responseText));
} else {
console.error('HTTP status error: ' + xhr.status);
}
}
};
5. Working with Request Headers
Request headers can be customized to provide additional information to the server or retrieve specific response headers:
xhr.setRequestHeader('Authorization', 'Bearer ' + authToken);
console.log(xhr.getResponseHeader('Content-Type'));
In this example, the 'Authorization' header is set with an authentication token, and the 'Content-Type' response header is retrieved from the server.
6. Monitoring Request Progress
The onprogress
event listener can be used to monitor the progress of file uploads and downloads:
xhr.onprogress = function (event) {
if (event.lengthComputable) {
const progress = (event.loaded / event.total) * 100;
console.log('Progress: ' + progress + '%');
}
};
7. Aborting Requests
The abort()
method can be used to cancel ongoing requests, providing a better user experience when necessary:
xhr.abort();
Developers should handle request cancellation gracefully within their applications.
Advanced Techniques and Best Practices
Utilizing Promises and Async/Await
Using Promises and async/await can lead to cleaner and more maintainable code:
function fetchData(url) {
return new Promise((resolve, reject) => {
const xhr = new XMLHttpRequest();
xhr.open('GET', url);
xhr.onload = () => resolve(JSON.parse(xhr.responseText));
xhr.onerror = () => reject(xhr.statusText);
xhr.send();
});
}
async function getData() {
try {
const data = await fetchData('<https://api.example.com/data>');
console.log(data);
} catch (error) {
console.error(error);
}
}
getData();
Optimizing Performance
Implementing caching strategies and throttling or debouncing requests can improve performance and reduce server load:
xhr.setRequestHeader('Cache-Control', 'max-age=3600'); // Cache the response for 1 hour
Security Considerations
Developers should validate input and output data and protect against Cross-Site Request Forgery (CSRF) attacks:
xhr.setRequestHeader('X-CSRF-Token', csrfToken);
Conclusion
Mastering XMLHttpRequest techniques is vital for JavaScript developers to create efficient and responsive web applications. By understanding and implementing these techniques, developers can ensure smooth communication with servers and improve the overall user experience. While XMLHttpRequest is a powerful tool, developers may also explore alternative techniques and libraries such as the Fetch API and Axios for additional features and improved convenience.
Frequently Asked Questions
What is the difference between XMLHttpRequest and the Fetch API?
XMLHttpRequest is an older API for making network requests in JavaScript, while the Fetch API is a more modern alternative. The Fetch API is built on Promises, providing a more convenient and cleaner syntax. However, XMLHttpRequest is more widely supported in older browsers.
Can I use XMLHttpRequest to make cross-domain requests?
Yes, you can use XMLHttpRequest to make cross-domain requests, but you may run into CORS (Cross-Origin Resource Sharing) issues. To resolve these issues, the server must be configured to allow cross-domain requests from your web application's domain.
How do I handle file uploads with XMLHttpRequest?
To handle file uploads with XMLHttpRequest, you can use the FormData API to create a multipart form request and attach the file to the request payload.
What is the maximum size of data that can be sent using XMLHttpRequest?
There is no specific maximum size for data sent using XMLHttpRequest, but performance may degrade for very large payloads. It is recommended to use streaming APIs or divide large payloads into smaller chunks when sending large amounts of data.
Can I use XMLHttpRequest with other data formats besides XML and JSON?
Yes, XMLHttpRequest can be used to send and receive data in various formats, including plain text, XML, JSON, and binary data (e.g., images and files). The appropriate request and response headers must be set for the specific data format being used.