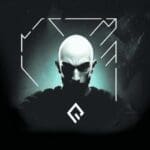
Wordpress REST API - Connect CMS Data To External Applications
In today's fast-paced world, integrating applications and sharing data across platforms has become an essential aspect of web development. Wordpress, as a leading content management system (CMS), provides a powerful tool called the Wordpress REST API that allows developers to connect CMS data to external applications. In this article, we will dive deep into the Wordpress REST API, its functionalities, and how to use it effectively.
Introduction
The Wordpress REST API is a feature introduced in Wordpress 4.7 that enables developers to interact with the CMS data through HTTP requests. This means that you can perform CRUD (Create, Read, Update, and Delete) operations on your website's content programmatically. Connecting CMS data to external applications can lead to a myriad of possibilities, such as mobile app development, remote content management, and data visualization, among others.
Getting Started with Wordpress REST API
Enabling the REST API
For Wordpress installations version 4.7 and above, the REST API is enabled by default. If you are using an older version of Wordpress, you will need to install the WP REST API plugin to enable the functionality.
Accessing the REST API
To access the REST API, make a GET request to the /wp-json/wp/v2/
endpoint. This will return a list of available routes and endpoints that you can use to interact with your Wordpress data.
For instance, to get a list of your site's posts, you can send a GET request to /wp-json/wp/v2/posts
.
You can explore the available endpoints and schema by visiting the Wordpress REST API Handbook.
Authentication
To perform CRUD operations on protected resources, such as creating or updating posts, you need to authenticate your requests. There are three primary authentication methods:
Cookie Authentication
Cookie authentication is the recommended method for plugins and themes, as it ensures that the user is already authenticated in Wordpress. To use cookie authentication, you need to include a nonce in your API request. A nonce is a unique token generated by Wordpress that ensures the request is valid and secure.
Here's an example of using the jQuery AJAX method to send an authenticated request:
javascript
$.ajax({
url: '/wp-json/wp/v2/posts',
method: 'POST',
data: {
title: 'My New Post',
content: 'This is the content of my new post.'
},
beforeSend: function(xhr) {
xhr.setRequestHeader('X-WP-Nonce', yourNonceValue);
}
});
To generate a nonce, use the wp_create_nonce()
function in your PHP code:
php
$nonce = wp_create_nonce('wp_rest');
OAuth 1.0a Authentication
OAuth 1.0a authentication is recommended for external applications that need to access your Wordpress data. To use OAuth 1.0a, you need to install the WP REST API - OAuth 1.0a Server plugin.
Once the plugin is installed, you can create a client application by following these steps:
- Go to
Settings
>REST API OAuth1
in your Wordpress admin panel. - Click on
Add New Client
. - Fill in the required fields and click on
Save Client
.
After creating the client, you will receive the client key and client secret, which you can use to authenticate your requests.
Basic Authentication
Basic authentication is suitable for development and testing purposes but is not recommended for production due to security concerns. To use basic authentication, you need to install the Basic Auth plugin
With the Basic Auth plugin installed, you can authenticate your requests by including the Authorization
header with your username and password encoded in base64 format.
Here's an example using the fetch
method in JavaScript:
javascript
fetch('/wp-json/wp/v2/posts', {
method: 'POST',
headers: {
'Authorization': 'Basic ' + btoa('username:password'),
'Content-Type': 'application/json'
},
body: JSON.stringify({
title: 'My New Post',
content: 'This is the content of my new post.'
})
});
Remember that basic authentication should only be used in secure environments, and you must use HTTPS to protect your credentials during transmission.
CRUD Operations
Now that we have covered authentication methods, let's dive into CRUD operations with the Wordpress REST API.
Creating a Post
To create a new post, send a POST request to /wp-json/wp/v2/posts
. You need to include the required fields, such as title
, content
, and status
, as parameters in the request body.
Here's an example using the fetch
method in JavaScript:
javascript
fetch('/wp-json/wp/v2/posts', {
method: 'POST',
headers: {
'Authorization': 'Bearer ' + yourAccessToken,
'Content-Type': 'application/json'
},
body: JSON.stringify({
title: 'My New Post',
content: 'This is the content of my new post.',
status: 'publish'
})
});
Retrieving Posts
To retrieve a list of posts, send a GET request to /wp-json/wp/v2/posts
. You can also use various query parameters to filter and sort the results, such as categories
, tags
, orderby
, and order
.
For example, to get the five most recent posts in the "News" category, you can use the following request:
javascript
fetch('/wp-json/wp/v2/posts?categories=news&per_page=5&orderby=date&order=desc')
.then(response => response.json())
.then(posts => console.log(posts));
Updating a Post
To update an existing post, send a PUT or PATCH request to /wp-json/wp/v2/posts/<id>
. Include the fields you want to update as parameters in the request body.
Here's an example of updating the title and content of a post using the fetch
method in JavaScript:
javascript
fetch('/wp-json/wp/v2/posts/1', {
method: 'PUT',
headers: {
'Authorization': 'Bearer ' + yourAccessToken,
'Content-Type': 'application/json'
},
body: JSON.stringify({
title: 'Updated Post Title',
content: 'This is the updated content of my post.'
})
});
Deleting a Post
To delete a post, send a DELETE request to /wp-json/wp/v2/posts/<id>
. By default, this will move the post to the trash. If you want to permanently delete the post, include the force=true
query parameter in the request.
Here's an example using the fetch
method in JavaScript:
javascript
fetch('/wp-json/wp/v2/posts/1?force=true', {
method: 'DELETE',
headers: {
'Authorization': 'Bearer ' + yourAccessToken
}
});
Working with Custom Post Types
If you have custom post types registered on your Wordpress site, you can also use the REST API to interact with them.
Registering a Custom Post Type
To make your custom post type available via the REST API, you need to add the
'show_in_rest' => true
argument when registering the post type using the register_post_type()
function in your PHP code. Additionally, you can specify the base path for your custom post type's REST API endpoints using the 'rest_base'
argument.
Here's an example of registering a custom post type called "portfolio":
php
function register_portfolio_post_type() {
$args = array(
'public' => true,
'label' => 'Portfolio',
'show_in_rest' => true,
'rest_base' => 'portfolio'
);
register_post_type('portfolio', $args);
}
add_action('init', 'register_portfolio_post_type');
CRUD Operations for Custom Post Types
Once your custom post type is registered and exposed to the REST API, you can interact with it using the same CRUD operations as standard posts. Simply replace the /wp-json/wp/v2/posts
endpoint with your custom post type's REST base.
For example, to retrieve a list of portfolio items, send a GET request to /wp-json/wp/v2/portfolio
.
Advanced Usage
The Wordpress REST API is highly extensible, allowing you to create custom routes and endpoints, work with meta data, and manage media files.
Extending the REST API
To create custom routes and endpoints, you can use the register_rest_route()
function in your PHP code. This allows you to define custom logic for handling requests to your custom endpoints.
Here's an example of creating a custom endpoint that returns the five most recent posts from the "News" category:
php
function get_latest_news_posts(WP_REST_Request $request) {
$args = array(
'post_type' => 'post',
'category_name' => 'news',
'posts_per_page' => 5,
'orderby' => 'date',
'order' => 'DESC'
);
$posts = get_posts($args);
return new WP_REST_Response($posts, 200);
}
function register_latest_news_route() {
register_rest_route('my-plugin/v1', '/latest-news', array(
'methods' => 'GET',
'callback' => 'get_latest_news_posts'
));
}
add_action('rest_api_init', 'register_latest_news_route');
Working with Meta Data
To interact with meta data using the REST API, you first need to register your custom meta fields using the register_meta()
function in your PHP code. This will expose your meta fields to the REST API and allow you to perform CRUD operations on them.
Here's an example of registering a custom meta field called "client_name" for the "portfolio" post type:
php
function register_client_name_meta() {
register_meta('post', 'client_name', array(
'show_in_rest' => true,
'single' => true,
'type' => 'string'
));
}
add_action('init', 'register_client_name_meta');
Managing Media
You can use the REST API to upload and manage media files in your Wordpress site. To upload a new media file, send a POST request to /wp-json/wp/v2/media
, including the file as a binary attachment and specifying the Content-Disposition
and Content-Type
headers.
Once the media file is uploaded, you can associate it with a post using the featured_media
parameter when creating or updating the post.
Here's an example of uploading a media file and associating it with a post using JavaScript:
javascript
async function uploadMediaFile(file) {
const mediaResponse = await fetch('/wp-json/wp/v2/media', {
method: 'POST',
headers: {
'Authorization': '
Bearer ' + yourAccessToken,
'Content-Disposition': attachment; filename="${file.name}",
'Content-Type': file.type
},
body: file
});
const media = await mediaResponse.json();
return media.id;
}
async function createPostWithFeaturedImage(title, content, mediaId) {
const postResponse = await fetch('/wp-json/wp/v2/posts', {
method: 'POST',
headers: {
'Authorization': 'Bearer ' + yourAccessToken,
'Content-Type': 'application/json'
},
body: JSON.stringify({
title: title,
content: content,
status: 'publish',
featured_media: mediaId
})
});
const post = await postResponse.json();
return post;
}
// Usage example
const fileInput = document.getElementById('file-input');
fileInput.addEventListener('change', async (event) => {
const file = event.target.files[0];
const mediaId = await uploadMediaFile(file);
const post = await createPostWithFeaturedImage('My Post with Featured Image', 'This is the content of my post.', mediaId);
console.log(post);
});
Security and Performance
When working with the Wordpress REST API, it's crucial to keep security and performance in mind.
Securing Your API
To prevent unauthorized access to your API, you can limit access to specific routes and implement Cross-Origin Resource Sharing (CORS) and rate limiting. You can use plugins like WP REST API Controller and WP REST API Log to help manage your API's security.
Optimizing Performance
To ensure your API performs well, you can reduce the response payload by only requesting the fields you need using the _fields
query parameter. Additionally, you can utilize caching strategies, such as server-side caching and browser caching, to reduce the load on your server and improve response times.
For example, to retrieve only the title and date of posts, send a GET request to /wp-json/wp/v2/posts?_fields=title,date
.
Conclusion
In this article, we covered the Wordpress REST API, how to enable and access it, various authentication methods, CRUD operations, working with custom post types, advanced usage, and security and performance considerations. By connecting your CMS data to external applications, you can unlock a world of possibilities and create unique, engaging, and interactive experiences for your users.
With a solid understanding of the Wordpress REST API, you can leverage its power to build innovative and dynamic applications that integrate seamlessly with your Wordpress website.
Frequently Asked Questions
How do I enable the Wordpress REST API on my website?
The Wordpress REST API is enabled by default on Wordpress websites running version 4.7 or later. If you have a custom installation or a plugin that disables the REST API, you can enable it by removing any filters or actions that disable the REST API in your theme's functions.php
file or by deactivating the plugin.
Can I use the Wordpress REST API with custom post types?
Yes, you can use the Wordpress REST API with custom post types. To make a custom post type available via the REST API, you need to add the 'show_in_rest' => true
argument when registering the post type using the register_post_type()
function in your PHP code. You can also specify the base path for your custom post type's REST API endpoints using the 'rest_base'
argument.
What are the performance implications of using the Wordpress REST API?
Using the Wordpress REST API can have an impact on your website's performance, especially if you make a large number of requests or request large amounts of data. To optimize performance, reduce the response payload by only requesting the fields you need using the _fields
query parameter, and implement caching strategies such as server-side caching and browser caching.
How do I secure my Wordpress REST API?
To secure your Wordpress REST API, limit access to specific routes, implement Cross-Origin Resource Sharing (CORS), and use rate limiting. Use secure authentication methods, such as OAuth 2.0, and ensure that your API requests are made over HTTPS. You can also use plugins like WP REST API Controller and WP REST API Log to help manage your API's security.
Can I extend the Wordpress REST API with custom routes and endpoints?
Yes, you can extend the Wordpress REST API with custom routes and endpoints by using the register_rest_route()
function in your PHP code. This allows you to define custom logic for handling requests to your custom endpoints and create bespoke functionality tailored to your specific needs.