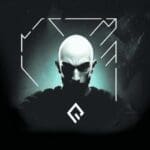
Introduction
Web Components are a collection of web platform technologies that allow developers to create reusable, encapsulated, and modular components for the web. By leveraging these technologies, you can build custom elements that extend existing HTML elements or define entirely new ones. These components can be easily shared and used in various projects, simplifying the development process and promoting code reusability.
In this article, we'll explore the three main technologies that make up Web Components: Custom Elements, Shadow DOM, and HTML Templates. We'll examine how these technologies work together to provide a powerful and flexible way of creating complex user interfaces.
Custom Elements
What are Custom Elements?
Custom Elements are a core part of the Web Components specification. They allow you to create your own custom HTML elements that can be used alongside the existing standard HTML elements. Custom Elements enable developers to build reusable and encapsulated components that can be easily integrated into any web application.
By creating Custom Elements, you can define new HTML tags that encapsulate functionality, styles, and markup. This encapsulation ensures that your custom elements can be easily reused across multiple projects without having to worry about styles or scripts conflicting with the rest of your application.
Creating a Custom Element
To create a Custom Element, you need to perform the following steps:
- Extend the
HTMLElement
class: In order to create a new custom element, you need to define a new class that extends the built-inHTMLElement
class. This new class will inherit all the properties and methods of the standard HTML elements and can be extended with your own custom functionality.class MyCustomElement extends HTMLElement { // Your custom functionality goes here }
- Register the custom element with
customElements.define()
: Once you've defined your custom element class, you need to register it with the browser. To do this, you'll use thecustomElements.define()
method, passing in the new element's tag name and the class that represents it.customElements.define('my-custom-element', MyCustomElement);
- Using lifecycle callbacks: Custom Elements can respond to various lifecycle events, such as when they are added or removed from the DOM. By implementing methods like
connectedCallback
,disconnectedCallback
,attributeChangedCallback
, andadoptedCallback
, you can add custom behavior to your elements at specific points in their lifecycle.class MyCustomElement extends HTMLElement { connectedCallback() { console.log('My custom element has been added to the DOM'); } }
Using Custom Elements
Once you've created and registered your custom element, you can start using it in your HTML markup. To include a custom element, simply use the tag name you registered with customElements.define()
:
<my-custom-element></my-custom-element>
You can also interact with custom elements using JavaScript, just like any other HTML element. For example, you can create instances of your custom element using the document.createElement()
method, or access them using document.querySelector()
:
// Create a new instance of MyCustomElement
const myElement = document.createElement('my-custom-element');
// Add the custom element to the DOM
document.body.appendChild(myElement);
// Access an existing custom element in the DOM
const existingElement = document.querySelector('my-custom-element');
Shadow DOM
What is Shadow DOM?
Shadow DOM is another key technology in the Web Components specification, providing a way to encapsulate styles and markup within a custom element. This encapsulation ensures that the internal structure, styles, and behaviors of a component are isolated from the rest of the document. This isolation prevents styles and scripts from unintentionally affecting other parts of your application and makes it easier to create reusable components.
In essence, Shadow DOM creates a separate DOM subtree for your custom element, which is shielded from the main document. This allows you to define internal styles and markup that won't leak out, and external styles and scripts won't interfere with the component.
Creating a Shadow DOM
To create a Shadow DOM for your custom element, follow these steps:
- Attach a shadow root with
attachShadow()
: Inside your custom element class, you need to create a shadow root by calling theattachShadow()
method. This method returns a reference to the shadow root, which you can use to add content to the Shadow DOM.class MyCustomElement extends HTMLElement { constructor() { super(); this.attachShadow({ mode: 'open' }); } }
TheattachShadow()
method accepts an options object with amode
property, which can be set to either'open'
or'closed'
. An open shadow root can be accessed from outside the component using theelement.shadowRoot
property, while a closed shadow root cannot be accessed directly from JavaScript. - Add content to the shadow root: Once you have a reference to the shadow root, you can start adding content to the Shadow DOM. You can use the standard DOM manipulation methods, such as
appendChild()
orinsertAdjacentHTML()
, to add elements, text, or HTML markup to the shadow root.class MyCustomElement extends HTMLElement { constructor() { super(); this.shadowRoot = this.attachShadow({ mode: 'open' }); this.shadowRoot.innerHTML = ` <h1>Hello, Shadow DOM!</h1> <p>This content is inside the shadow root.</p> `; } }
Styling Shadow DOM
When working with Shadow DOM, you can define styles that are scoped to the component, meaning they won't affect the rest of the document. There are two main ways to apply styles to the Shadow DOM:
- Applying internal styles to the shadow root: You can include a
<style>
element within the shadow root to apply styles that are scoped to the component. These styles will only affect the elements inside the Shadow DOM and won't leak out to the main document.class MyCustomElement extends HTMLElement { constructor() { super(); this.shadowRoot = this.attachShadow({ mode: 'open' }); this.shadowRoot.innerHTML = ` <style> h1 { color: red; } </style> <h1>Hello, Shadow DOM!</h1> <p>This content is inside the shadow root.</p> `; } }
- Using CSS custom properties for external styling: If you want to allow external styles to affect certain parts of your component, you can use CSS custom properties (also known as CSS variables). This way, the component's styles can be controlled from outside the Shadow DOM.
class MyCustomElement extends HTMLElement { constructor() { super(); this.shadowRoot = this.attachShadow({ mode: 'open' }); this.shadowRoot.innerHTML = ` <style> h1 { color: var(--header-color, red); } </style> <h1>Hello, Shadow DOM!</h1> <p>This content is inside the shadow root.</p> `; } }
In this example, the--header-color
custom property is used to control the color of the<h1>
element. If the property is not set, the default value ofred
will be used.
Slots and Composition
Shadow DOM provides a powerful feature called slots, which allows you to project content from the main document into your custom element. Slots are created using the <slot>
element and can be used to define placeholders for external content within the Shadow DOM.
There are two types of slots: default slots and named slots. Default slots will receive any content that is not explicitly assigned to a named slot. Named slots are created by setting the name
attribute on a <slot>
element and can be targeted by setting the slot
attribute on the content to be projected.
Here's an example of using slots in a custom element:
class MyCustomElement extends HTMLElement {
constructor() {
super();
this.shadowRoot = this.attachShadow({ mode: 'open' });
this.shadowRoot.innerHTML = `<style> h1 { color: var(--header-color, red); } </style> <h1><slot name="header">Default Header</slot></h1> <p><slot>Default content</slot></p>` ;
}
}
customElements.define('my-custom-element', MyCustomElement);
In this example, two <slot>
elements are used to define the placeholders for the header and content. The header slot is a named slot with the name header
, while the content slot is a default slot. If no content is assigned to these slots, the default values will be used.
To use the slots in your HTML markup, you can add content inside the custom element and assign it to the appropriate slot using the slot
attribute:
```html
<my-custom-element>
<span slot="header">Hello, Slots!</span>
This content will go into the default slot.
</my-custom-element>
In this case, the header slot will display "Hello, Slots!" instead of the default header, and the content slot will display the text inside the custom element.
HTML Templates
What are HTML Templates?
HTML Templates are a way to define reusable chunks of markup that can be cloned and inserted into a document at runtime. They are a fundamental part of the Web Components ecosystem, enabling the creation of reusable and encapsulated components.
HTML Templates are created using the <template>
element, which allows you to define a piece of markup that is not rendered by the browser until it is explicitly cloned and added to the DOM. This makes templates an efficient way to create and manage content that is not immediately visible or used multiple times on a page.
Using HTML Templates
To use an HTML template, you need to perform the following steps:
- Cloning and importing template content with
importNode()
: To use the content of a template, you need to first clone it using theimportNode()
method. This method creates a deep copy of the template's content and allows you to import it into another document or a Shadow DOM.const template = document.querySelector('#my-template'); const content = document.importNode(template.content, true);
TheimportNode()
method takes two arguments: the node to be imported and a boolean indicating whether to perform a deep clone. If the boolean is set totrue
, the entire subtree of the node will be cloned; otherwise, only the node itself will be cloned. - Appending template content to the DOM: Once you have cloned the template content, you can append it to the DOM or a Shadow DOM using standard DOM manipulation methods, such as
appendChild()
orinsertBefore()
.const container = document.querySelector('#container'); container.appendChild(content);
In this example, the cloned template content is appended to a container element in the main document. If you were using a custom element with Shadow DOM, you would append the content to the shadow root instead.
By combining HTML Templates with Custom Elements and Shadow DOM, you can create powerful, reusable, and encapsulated components for your web applications.
Putting It All Together: Building a Web Component
Now that we've explored Custom Elements, Shadow DOM, and HTML Templates individually, let's combine them to create a sample web component with encapsulated functionality.
In this example, we'll create a simple "user-card" component that displays a user's name, email, and profile picture.
First, let's define the HTML template for the user card:
<template id="user-card-template">
<style>
:host {
display: block;
border: 1px solid #ccc;
border-radius: 4px;
padding: 16px;
font-family: sans-serif;
}
.profile-image {
width: 48px;
height: 48px;
border-radius: 50%;
margin-right: 16px;
}
.user-info {
display: flex;
align-items: center;
}
.user-details {
line-height: 1.5;
}
</style>
<div class="user-info">
<img class="profile-image" />
<div class="user-details">
<h3 class="name"></h3>
<p class="email"></p>
</div>
</div>
</template>
Now, let's create the UserCard
custom element that extends HTMLElement
and uses the template:
class UserCard extends HTMLElement {
constructor() {
super();
const template = document.querySelector('#user-card-template');
const content = document.importNode(template.content, true);
this.attachShadow({ mode: 'open' });
this.shadowRoot.appendChild(content);
}
connectedCallback() {
this.shadowRoot.querySelector('.profile-image').src = this.getAttribute('image');
this.shadowRoot.querySelector('.name').textContent = this.getAttribute('name');
this.shadowRoot.querySelector('.email').textContent = this.getAttribute('email');
}
}
customElements.define('user-card', UserCard);
In this example, we've created a UserCard
custom element that uses the previously defined HTML template. Inside the constructor
, we import the template content and append it to the shadow root. The connectedCallback
lifecycle method is used to populate the user information using the attributes passed to the custom element.
To use the user-card
component, simply include it in your HTML markup and provide the necessary attributes:
<user-card
name="Jane Doe"
email="[email protected]"
image="<https://example.com/jane-doe.jpg>"
></user-card>
This will create a user card with the provided name, email, and profile picture, all encapsulated within a single custom element.
Conclusion
In this article, we've explored Web Components and the core technologies that make them possible: Custom Elements, Shadow DOM, and HTML Templates. By combining these technologies, you can create powerful, reusable, and encapsulated components for your web applications.
We encourage you to experiment with these technologies and start building your own web components. Whether you're creating a simple UI component or a complex application, Web Components can help streamline your development process, promote code reusability, and improve the overall maintainability of your projects.
Frequently Asked Questions
1. Are Web Components supported in all modern browsers?
Yes, Web Components are supported in all modern browsers, including Chrome, Firefox, Safari, and Edge. However, older versions of Internet Explorer (IE) do not support Web Components natively. If you need to support older browsers, you can use polyfills like the webcomponents.js library to enable Web Components functionality.
2. Can I use Web Components with popular front-end frameworks like React, Angular, or Vue?
Yes, Web Components can be used in conjunction with popular front-end frameworks like React, Angular, or Vue. These frameworks can interact with Web Components just like any other HTML element. However, some additional configuration or wrapper components may be required to ensure seamless integration, especially when it comes to handling properties, events, or slots.
3. How do Web Components compare to framework-specific components?
Web Components are native to the web platform and do not rely on any specific framework or library. This makes them more portable and reusable across different projects and frameworks. However, framework-specific components often provide additional features, optimizations, and tooling that are tailored to the specific framework.
Ultimately, the choice between Web Components and framework-specific components depends on your project requirements, technology stack, and personal preferences.
4. What are the best practices for creating Web Components?
Some best practices for creating Web Components include:
- Use semantic and descriptive tag names for your custom elements to convey their purpose and functionality.
- Make your components self-contained and encapsulate styles, markup, and behavior within the component using Shadow DOM.
- Provide a clear and easy-to-use API for your components, exposing properties, methods, and events as needed.
- Consider accessibility when designing and implementing your components, ensuring they can be used by all users, including those with disabilities.
5. Can I use third-party libraries and CSS frameworks with Web Components?
Yes, you can use third-party libraries and CSS frameworks with Web Components. However, keep in mind that encapsulation provided by Shadow DOM may require additional configuration or workarounds to ensure styles and scripts are applied correctly.
For example, to use a CSS framework like Bootstrap within a Web Component, you would need to manually include the relevant styles within the component's Shadow DOM. Alternatively, you can use CSS custom properties or @import
statements to include external stylesheets.
When it comes to JavaScript libraries, you can use them as you would with any other HTML element, either by including the library in your main document or by importing it as a module within your custom element's JavaScript code.