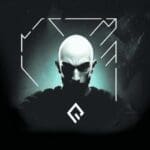
Sveltekit - How To Build Web Applications With Svelte
Introduction
Svelte is a JavaScript-based web application framework that has gained popularity among web developers in recent years. One of the newest additions to the Svelte ecosystem is SvelteKit, a framework for building web applications using Svelte.
In this article, we'll explore what SvelteKit is, how it works, and how to build web applications using it. We'll cover everything from setting up a SvelteKit project to implementing routing, handling state, and working with server-side rendering and static site generation.
What is Svelte?
Before we dive into SvelteKit, let's take a moment to understand what Svelte is and how it works. Svelte is a compile-time framework that generates vanilla JavaScript code. This means that Svelte takes your components and turns them into optimized JavaScript code that can be run in any browser.
One of the key features of Svelte is its reactive programming model. This means that when data changes in your application, Svelte automatically updates the UI to reflect those changes. This is achieved through a process called "reactive declarations," which allow you to declaratively define how your components should respond to changes in data.
Another key feature of Svelte is its component-based architecture. Components are reusable, self-contained units of code that can be easily composed together to create complex user interfaces.
What is SvelteKit?
SvelteKit is a framework for building web applications using Svelte. It provides a set of tools and conventions for creating Svelte-based web applications with features like built-in routing, server-side rendering (SSR), and static site generation (SSG).
One of the benefits of using SvelteKit is that it leverages the power of Svelte's reactive programming model and component-based architecture to create web applications that are fast and efficient.
SvelteKit also provides a set of APIs for working with APIs, database connections, and other backend functionality. This makes it easy to create full-stack web applications using Svelte and SvelteKit.
Setting Up a SvelteKit Project
The first step in building a web application with SvelteKit is to set up a new project. To do this, you'll need to have Node.js and npm installed on your machine.
Once you have Node.js and npm installed, you can create a new SvelteKit project using the npm init svelte@next
command. This will create a new SvelteKit project in the current directory.
npm init svelte@next my-sveltekit-app
cd my-sveltekit-app
Once the project is set up, you can start the development server using the npm run dev
command.
npm run dev
This will start the development server and open a browser window with your new SvelteKit application.
Building Components in SvelteKit
One of the key features of Svelte is its component-based architecture. In SvelteKit, you can create Svelte components in the src/components
directory.
A Svelte component consists of HTML, CSS, and JavaScript code in a single .svelte
file. Here's an example of a simple Svelte component:
htm
<!-- MyComponent.svelte -->
<script>
export let name;
</script>
<h1>Hello {name}!</h1>
In this component, we've defined a single prop called name
. We can use this prop in our component's template by wrapping it in curly braces ({}
).
To use this component in our SvelteKit application, we can import it into our pages and pass in the name
prop.
html
<!-- Home.svelte -->
<script>
import MyComponent from '$components/MyComponent.svelte';
</script>
<MyComponent name="John" />
``
In this example, we've imported our MyComponent
component and passed in the name
prop with the value of "John". When we render the Home
page, the MyComponent
component will render with the value of name
set to "John".
Routing in SvelteKit
SvelteKit provides a built-in router that makes it easy to handle navigation in your web application. You can define your routes in the src/routes
directory using .svelte
or .js
files.
Routes in SvelteKit are defined using the load
function, which returns an object with properties for the page's data and the component to render. Here's an example of a simple route definition:
javascript
// src/routes/index.svelte
export async function load() {
return {
pageData: { title: 'Home' },
component: () => import('./Home.svelte')
};
}
In this example, we've defined a route for the home page that returns an object with the page data (title
) and the component to render (Home.svelte
).
To define dynamic routes that include parameters, you can use the :param
syntax in your route definitions. Here's an example of a dynamic route definition:
javascript
// src/routes/user/[id].svelte
export async function load({ params }) {
const { id } = params;
const userData = await fetch(`/api/users/${id}`);
const user = await userData.json();
return {
pageData: { title: `User ${id}` },
component: () => import('./User.svelte'),
props: { user }
};
}
In this example, we've defined a dynamic route for user pages that includes a parameter for the user's id
. We've also defined a load
function that fetches the user data from an API and passes it as a prop to the User
component.
Handling State in SvelteKit
SvelteKit provides a built-in store API that makes it easy to handle state in your web application. Stores are reactive data containers that can be used to manage data that needs to be shared across components.
To create a store in SvelteKit, you can use the writable
or readable
store functions. Here's an example of a simple writable store:
javascript
// src/stores/count.js
import { writable } from 'svelte/store';
export const count = writable(0);
In this example, we've defined a count
store that is initialized with a value of 0
. We can update the value of the store by calling its set
method.
To use the count
store in a Svelte component, we can import it and use the $
prefix to create a reactive binding to the store's value:
html
<!-- Counter.svelte -->
<script>
import { count } from '$lib/stores/count.js';
</script>
<button on:click={() => $count += 1}>Increment</button>
<p>Count: {$count}</p>
In this example, we've imported the count
store and created a reactive binding to its value using the $
prefix. When the user clicks the "Increment" button, the value of the count
store will be updated, and the UI will be automatically updated to reflect the new value.
Server-Side Rendering and Static Site Generation in SvelteKit
SvelteKit provides built-in support for server-side rendering (SSR) and static site generation (SSG), which can help improve the performance and SEO of your web application.
To enable SSR or SSG in your SvelteKit application, you can use the adapter
option in your svelte.config.js
file to configure your application for the appropriate deployment environment.
Server-Side Rendering
To enable SSR in your SvelteKit application, you can use the @sveltejs/adapter-node
adapter. This adapter allows you to deploy your application to a Node.js server that can generate HTML on the server and send it to the client.
Here's an example of how to configure your application for SSR using the @sveltejs/adapter-node
adapter:
javascript
// svelte.config.js
import node from '@sveltejs/adapter-node';
export default {
kit: {
adapter: node()
}
};
With SSR enabled, SvelteKit will automatically generate HTML on the server for each page in your application and send it to the client, which can help improve the performance of your web application by reducing the amount of client-side rendering required.
Static Site Generation
To enable SSG in your SvelteKit application, you can use the @sveltejs/adapter-static
adapter. This adapter allows you to generate a fully static version of your web application that can be hosted on a static file server.
Here's an example of how to configure your application for SSG using the @sveltejs/adapter-static
adapter:
javascript
// svelte.config.js
import staticAdapter from '@sveltejs/adapter-static';
export default {
kit: {
adapter: staticAdapter(),
target: '#svelte'
}
};
With SSG enabled, SvelteKit will generate a static version of your web application for each page in your application, which can help improve the performance and SEO of your web application by reducing the amount of server-side rendering and client-side rendering required.
Conclusion
SvelteKit is a powerful web framework that provides a modern and intuitive approach to building web applications. With its built-in routing, state management, and server-side rendering and static site generation capabilities, SvelteKit is a great choice for building fast, responsive, and scalable web applications.
Whether you're a seasoned web developer or just getting started, SvelteKit is a great choice for building modern web applications that deliver a great user experience. So why not give it a try today and see how SvelteKit can help you build better web applications, faster?
Frequently Asked Questions
What is SvelteKit Used For?
SvelteKit is a web application framework built on top of Svelte, a popular JavaScript library for building user interfaces. SvelteKit provides a comprehensive set of tools and features for building modern web applications, including built-in routing, state management, and server-side rendering and static site generation capabilities.
What are the benefits of using SvelteKit?
SvelteKit offers several benefits for building web applications, including:
- Fast and efficient rendering: SvelteKit compiles your application to highly optimized JavaScript, resulting in faster and more efficient rendering compared to other frameworks.
- Easy to learn and use: SvelteKit's simple and intuitive syntax and API make it easy for developers to get started and build web applications quickly.
- Built-in server-side rendering and static site generation: SvelteKit's built-in server-side rendering and static site generation capabilities make it easy to build fast and SEO-friendly web applications.
- Flexible and extensible: SvelteKit's modular architecture and plugin system make it easy to customize and extend the framework to meet your specific needs.
How does SvelteKit compare to other web frameworks like React and Vue?
SvelteKit differs from other web frameworks like React and Vue in several ways, including:
- Performance: SvelteKit's highly optimized rendering engine and compile-time optimizations result in faster and more efficient rendering compared to other frameworks.
- Ease of use: SvelteKit's simple and intuitive syntax and API make it easy for developers to get started and build web applications quickly.
- Server-side rendering and static site generation: SvelteKit's built-in server-side rendering and static site generation capabilities make it easy to build fast and SEO-friendly web applications.
- Developer experience: SvelteKit's modular architecture and plugin system provide a great developer experience and make it easy to customize and extend the framework.
How do I deploy a SvelteKit application?
SvelteKit applications can be deployed to a variety of hosting providers, including traditional web servers and cloud platforms like Vercel and Netlify. To deploy a SvelteKit application, you can follow these general steps:
- Build your SvelteKit application using the
npm run build
command. - Choose a hosting provider and configure your deployment environment.
- Deploy your application to your chosen hosting provider.
You may need to configure your SvelteKit application for your specific deployment environment using the adapter
option in your svelte.config.js
file.
Can I use SvelteKit with a database or API?
Yes, SvelteKit can be used with a variety of databases and APIs. SvelteKit provides built-in support for server-side rendering and static site generation, which can be used to fetch data from APIs or databases and render it on the server.
To use a database or API with SvelteKit, you can use a library like fetch
or axios
to make requests to your backend server and retrieve data. You can then use SvelteKit's built-in routing and state management capabilities to display the data on your web application.