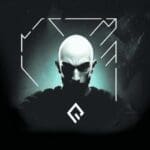
MessagePack vs Protobuf - Which Binary Data Framework Should You Use?
Introduction
MessagePack and Protocol Buffers (Protobuf) are two popular binary data frameworks that developers use for efficient data exchange and serialization. Choosing between these two frameworks depends on factors such as ease of use, performance, extensibility, and requirements of your project. This article will provide a comparison of MessagePack and Protobuf, highlighting their strengths and limitations, and offer guidance on how to choose the best framework for your needs.
MessagePack
Overview and Features
MessagePack is a binary serialization format designed for efficient data exchange between different systems. It is language-agnostic and offers support for a wide range of popular programming languages. MessagePack provides a compact binary representation of JSON-like data structures, which results in smaller message sizes and faster serialization and deserialization compared to JSON.
Strengths
- Simple and easy-to-use API, allowing for quick integration into projects
- Smaller message sizes compared to JSON, reducing data transfer overhead
- Faster serialization and deserialization than JSON, improving performance
Limitations
- Less feature-rich and extensible compared to Protobuf, which may limit its usefulness in complex projects
- Limited type checking and schema validation capabilities, potentially leading to runtime errors and data inconsistency
Protocol Buffers (Protobuf)
Overview and Features
Protocol Buffers, or Protobuf, is a language-neutral and platform-neutral data serialization framework developed by Google. It offers a compact binary format for efficient data transfer and supports schema evolution, ensuring backward compatibility as your data structures change over time. Protobuf also provides strong typing and schema validation capabilities, which helps maintain data consistency across systems.
Strengths
- Strong typing and schema validation, reducing the risk of data inconsistency and runtime errors
- Efficient serialization and deserialization, resulting in high-performance data exchange
- Extensible framework that supports custom options and extensions, allowing for greater flexibility in complex projects
Limitations
- More complex setup and integration process compared to MessagePack, which may present a steeper learning curve for developers
- Less human-readable compared to JSON, making it more challenging to debug and understand serialized data
Setting Up a Project with MessagePack
1. Install the MessagePack library
First, install the appropriate MessagePack library for your programming language. For example, for JavaScript, you can use npm to install the msgpack5
package:
npm install msgpack5
2. Serialize and deserialize data
Next, import the MessagePack library in your project and use it to serialize and deserialize your data. Here's an example using JavaScript and the msgpack5
package:
const msgpack = require('msgpack5')();
const data = {
name: 'John Doe',
age: 30,
email: '[email protected]'
};
// Serialize data
const serializedData = msgpack.encode(data);
// Deserialize data
const deserializedData = msgpack.decode(serializedData);
Setting Up a Project with Protobuf
1. Install the Protocol Buffers compiler
Download and install the Protocol Buffers compiler (protoc) for your platform from the official releases page.
2. Define your data schema
Create a .proto
file to define your data schema. For example, create a file named person.proto
with the following content:
syntax = "proto3";
message Person {
string name = 1;
int32 age = 2;
string email = 3;
}
3. Generate code for your programming language
Use the protoc
command to generate code for your chosen programming language. For example, to generate JavaScript code, run:
protoc --js_out=import_style=commonjs,binary:. person.proto
This command will generate a person_pb.js
file with the JavaScript code for serialization and deserialization.
4. Serialize and deserialize data
Import the generated code in your project and use it to serialize and deserialize your data. Here's an example using JavaScript and the person_pb.js
file:
const { Person } = require('./person_pb.js');
const person = new Person();
person.setName('John Doe');
person.setAge(30);
person.setEmail('[email protected]');
// Serialize data
const serializedData = person.serializeBinary();
// Deserialize data
const deserializedData = Person.deserializeBinary(serializedData);
Comparing MessagePack and Protobuf
Feature | MessagePack | Protobuf |
Ease of Use | Simple API | More complex setup |
Performance | Faster than JSON | High-performance |
Extensibility | Limited | Rich support for extensibility |
Schema Evolution | Limited | Strong support |
Language Support | Wide range | Extensive |
Human-readability | Less readable | Less readable |
Type Checking | Limited | Strong typing |
Schema Validation | Limited | Yes |
Browser Compatibility | Good | Good |
Integration with gRPC | Possible | Native support |
Ease of Use
- MessagePack: Offers a simple and easy-to-use API, enabling quick integration into projects
- Protobuf: Requires a more complex setup and integration process, potentially presenting a steeper learning curve for developers
Performance
- MessagePack: Provides faster serialization and deserialization compared to JSON, but generally slower than Protobuf
- Protobuf: Delivers high performance in both serialization and deserialization, making it suitable for performance-critical applications
Extensibility and Schema Evolution
- MessagePack: Offers limited support for schema evolution and extensibility, which may restrict its usefulness in complex projects
- Protobuf: Supports rich schema evolution, custom options, and extensions, providing greater flexibility for evolving data structures
Language and Platform Support
- MessagePack: Supports a wide range of programming languages, making it suitable for diverse projects
- Protobuf: Provides extensive language and platform support, including popular languages such as Java, C++, Python, and Go
Choosing the Right Binary Data Framework
Consider Your Project Requirements
When deciding between MessagePack and Protobuf, evaluate the trade-offs between simplicity and extensibility. Consider whether your project requires schema validation and strong typing, which Protobuf supports, or whether the simplicity of MessagePack's API is more valuable for your use case.
Evaluate Performance Needs
Analyze the performance requirements of your project and consider the potential impact of serialization and deserialization speed. If high performance is a priority, Protobuf may be a better choice due to its efficient serialization and deserialization.
Integration and Tooling
Examine the ease of integration and available tooling for each framework. Consider the learning curve for your team and whether the simplicity of MessagePack or the extensibility of Protobuf better aligns with your project goals.
Conclusion
Both MessagePack and Protobuf offer valuable features for binary data serialization and exchange. Choosing the right framework depends on factors such as ease of use, performance, extensibility, and project requirements. By carefully considering these factors and aligning your choice with your project needs and goals, you can select the best binary data framework to ensure efficient and reliable data exchange in your application.
Frequently Asked Questions
Can MessagePack or Protobuf replace JSON in web applications?
Both MessagePack and Protobuf can replace JSON in web applications for data serialization and exchange. However, it is essential to consider factors such as browser compatibility, human-readability, and the ease of integration with existing tools and libraries before making the switch.
How do MessagePack and Protobuf handle compression?
MessagePack and Protobuf both provide compact binary representations of data, resulting in smaller message sizes compared to JSON. However, they do not inherently include data compression algorithms. You can use additional compression libraries like zlib or gzip to compress the serialized data further if needed.
Do MessagePack and Protobuf support streaming?
Both MessagePack and Protobuf can be used with streaming protocols, such as gRPC, to enable efficient streaming of data between clients and servers. Streaming can help reduce latency and improve performance in applications with large data sets or real-time requirements.
What are some alternatives to MessagePack and Protobuf?
Other binary data serialization frameworks and formats include Apache Avro, Apache Thrift, BSON, and CBOR. Each of these alternatives has its strengths and weaknesses, and the choice depends on the specific requirements and goals of your project.
Are there any security concerns when using MessagePack or Protobuf?
When using binary data serialization frameworks like MessagePack or Protobuf, it is essential to validate and sanitize input data to prevent security vulnerabilities such as injection attacks. Additionally, consider using encryption for sensitive data and implementing proper access controls to protect your application.