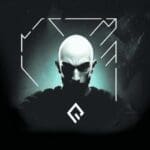
Introduction
In the world of programming languages and compilers, Abstract Syntax Trees (ASTs) and Parse Trees are two essential data structures that help us understand and analyze the structure and semantics of source code. While they might seem similar at first glance, there are some key differences between them that are important to understand. This article will provide a comprehensive comparison of ASTs and Parse Trees, exploring their definitions, characteristics, and applications. By understanding the differences between these two tree structures, you can make more informed decisions when working with programming languages and compiler construction.
Understanding Parse Trees
What are Parse Trees?
A Parse Tree, also known as a Concrete Syntax Tree or a Derivation Tree, is a tree data structure that represents the syntactic structure of a source code according to a formal grammar. The purpose of a Parse Tree is to explicitly represent the hierarchy and relationships between different syntactic elements in a program, as dictated by the grammar rules.
For example, consider the following simple arithmetic expression:
3 + 4 * 2
A Parse Tree for this expression would look like this:
Expr
/ \\\\
Term '+'
/ \\\\
Factor Expr
/ \\\\ / \\\\
'3' null Term null
/ \\\\
Factor '*'
/ \\\\
'4' Factor
/ \\\\
'2' null
In this Parse Tree, we can see how the expression is broken down into its constituent parts according to the grammar rules, with each node representing a syntactic element or token.
Characteristics of Parse Trees
Parse Trees have several distinctive characteristics, including:
- Derivation from formal grammar rules: Parse Trees are generated based on the grammar rules of a programming language. They provide a detailed representation of how the source code adheres to these rules.
- Inclusion of all tokens and syntactic elements: A Parse Tree includes every token and syntactic element present in the source code, making it a verbose and detailed representation of the code structure.
- Verbose and detailed representation of code structure: Due to their adherence to grammar rules and inclusion of all syntactic elements, Parse Trees can be quite complex and verbose, especially for large programs. This can make them harder to read and understand.
Understanding Abstract Syntax Trees (ASTs)
What are ASTs?
An Abstract Syntax Tree (AST) is another tree data structure used to represent the semantic structure of a source code. Unlike Parse Trees, ASTs focus on capturing the essential meaning of the code while abstracting away from the specific syntax and grammar rules. ASTs are a more concise and simplified representation of the source code structure, making them easier to work with for various applications such as code analysis, refactoring, and generation.
Using the same arithmetic expression as before (3 + 4 * 2
), the AST would look like this:
'+'
/ \\\\
'3' '*'
/ \\\\
'4' '2'
In the AST, we can see that the structure is simplified and more focused on the semantics of the expression.
Characteristics of ASTs
ASTs have several key characteristics, including:
- Abstraction from grammar rules: ASTs are not tied to the specific grammar rules of a programming language. Instead, they focus on capturing the essential meaning and structure of the source code.
- Exclusion of non-essential syntactic elements: ASTs only include the elements that are necessary for understanding the semantics of the code. This makes them a more concise representation of the code structure.
- Simplified and concise representation of code structure: Due to their abstraction from grammar rules and exclusion of non-essential elements, ASTs are generally simpler and easier to comprehend compared to Parse Trees.
Key Differences Between ASTs And Parse Trees
Representation of Code Structure
- Parse Trees: Provide a detailed and grammar-based representation of the source code structure. They adhere strictly to the grammar rules of a programming language, making them more complex and verbose.
- ASTs: Offer a simplified and semantics-focused representation of the code structure. They abstract away from grammar rules and include only the essential elements for understanding the code semantics, making them more concise and easier to read.
Elements Included
- Parse Trees: Include all tokens and syntactic elements present in the source code, providing a comprehensive view of the code structure.
- ASTs: Only include the essential elements necessary for understanding the semantics of the code, making them more focused and easier to work with.
Readability and Complexity
- Parse Trees: Tend to be more verbose and complex due to their adherence to grammar rules and inclusion of all syntactic elements.
- ASTs: Are generally simpler and easier to comprehend, as they abstract away from grammar rules and exclude non-essential syntactic elements.
Applications and Use Cases
- Parse Trees: Commonly used in syntax validation and compiler construction, as they provide a detailed view of the code structure and its adherence to grammar rules.
- ASTs: Widely used for code analysis, refactoring, generation, and transformation, as they offer a more concise and semantics-focused representation of the code structure.
Choosing Between ASTs And Parse Trees
Project Requirements and Goals
When deciding between using an AST or a Parse Tree for your project, it's important to consider the specific requirements and goals of the project. If you need a detailed representation of the code structure that adheres to grammar rules, a Parse Tree may be more suitable. However, if you're looking for a more concise and semantics-focused representation of the code, an AST might be the better choice.
Tooling and Libraries
There are various tools and libraries available for working with ASTs and Parse Trees. Some popular ones include ANTLR for generating parsers and Parse Trees, and Esprima for generating ASTs for JavaScript code. When choosing between ASTs and Parse Trees, consider the available tools and libraries that support your specific programming language and use case.
Performance and Scalability Considerations
The complexity and verbosity of Parse Trees can potentially impact performance and scalability, especially for large programs. On the other hand, ASTs are generally simpler and more concise, making them easier to work with and potentially leading to better performance. It's essential to assess the trade-offs between complexity and ease of use when deciding between ASTs and Parse Trees.
Conclusion
Understanding the key differences between ASTs and Parse Trees is crucial for various applications in programming languages and compiler construction. While Parse Trees provide a detailed and grammar-based representation of the code structure, ASTs offer a more simplified and semantics-focused view. By considering factors such as project requirements, available tools and libraries, and performance considerations, you can make an informed decision when choosing between these two tree structures.
Frequently Asked Questions
What is the main difference between ASTs and Parse Trees?
The main difference between ASTs and Parse Trees is their focus and representation of the source code structure. Parse Trees provide a detailed and grammar-based representation, while ASTs offer a simplified and semantics-focused view. This makes ASTs generally easier to work with and understand compared to Parse Trees.
Can ASTs and Parse Trees be used together?
Yes, ASTs and Parse Trees can be used together in certain scenarios. For example, in the process of compiler construction, a Parse Tree can be generated first to validate the syntax of the source code. Then, the Parse Tree can be transformed into an AST for further analysis, optimization, and code generation.
Which is more efficient: ASTs or Parse Trees?
In terms of efficiency, ASTs are generally considered to be more efficient due to their simplified and concise representation of the code structure. This makes them easier to work with and can potentially lead to better performance, especially for large programs.
Are there any tools that can generate both ASTs and Parse Trees?
Yes, there are tools like ANTLR that can generate both ASTs and Parse Trees for various programming languages. These tools can be helpful when working with different aspects of programming languages and compiler construction, as they provide the flexibility to choose between the two tree structures based on your specific needs.
Why are ASTs more popular than Parse Trees for code analysis and transformation?
ASTs are more popular for code analysis and transformation because they provide a more concise and semantics-focused representation of the code structure. This makes them easier to work with, understand, and manipulate, making them a better choice for applications like code analysis, refactoring, and generation.