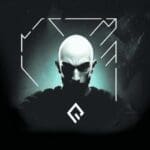
Introduction
Optimizing your website for production involves multiple steps, and one of the most important is minifying your CSS files. Minification is the process of removing unnecessary characters from code without affecting its functionality. Minifying Tailwind CSS can significantly reduce the size of your CSS files, leading to faster page load times and better performance. In this article, we'll discuss various techniques and tools to minify Tailwind CSS for production readiness, as well as some best practices to maintain a production-ready codebase.
Tailwind CSS Basics
What is Tailwind CSS
Tailwind CSS is a utility-first CSS framework that provides a set of pre-built classes for styling your web applications. It promotes a component-driven approach, allowing you to create custom designs with minimal CSS. Its flexibility and ease of use have made it popular among web developers.
Installing Tailwind CSS
You can install Tailwind CSS via npm or yarn by running the following commands:
npm install tailwindcss
# or
yarn add tailwindcss
Once installed, create a configuration file tailwind.config.js
in your project root directory. This file allows you to customize your Tailwind CSS configuration and add any additional plugins.
Minification Techniques
Using PurgeCSS
PurgeCSS is a tool that removes unused CSS from your files, significantly reducing their size. It's particularly useful for Tailwind CSS projects because the framework generates a large number of utility classes, many of which may not be used in your application.
To configure PurgeCSS with Tailwind CSS, you need to update your tailwind.config.js
file:
javascript
module.exports = {
purge: [
// Add the paths to your template files here
'./src/**/*.html',
'./src/**/*.vue',
'./src/**/*.jsx',
],
theme: {},
variants: {},
plugins: [],
}
CSSnano
CSSnano is a modular minifier for CSS that compresses and optimizes your code. It can be easily integrated with Tailwind CSS to further reduce the size of your CSS files.
To add CSSnano to your project, first, install it using npm or yarn:
npm install cssnano
# or
yarn add cssnano
Next, integrate it with your build process, which we'll discuss in the following sections.
Build Tools for Minification
Webpack
Webpack is a popular JavaScript module bundler that can be used to minify Tailwind CSS. To get started, first, install Webpack and its required loaders and plugins:
npm install webpack webpack-cli css-loader mini-css-extract-plugin postcss-loader purgecss-webpack-plugin cssnano
# or
yarn add webpack webpack-cli css-loader mini-css-extract-plugin postcss-loader purgecss-webpack-plugin cssnano
Then, create a webpack.config.js
file in your project root and add the following configuration:
javascript
const MiniCssExtractPlugin = require('mini-css-extract-plugin');
const PurgeCSSPlugin = require('purgecss-webpack-plugin');
const Cssnano = require('cssnano');
const path = require('path');
module.exports = {
mode: 'production',
entry: './src/index.js',
output: {
filename: 'bundle.js',
path: path.resolve(__dirname, 'dist'),
},
module: {
rules: [
{
test: /.css$/,
use: [
MiniCssExtractPlugin.loader,
'css-loader',
{
loader: 'postcss-loader',
options: {
postcssOptions: {
plugins: [
require('tailwindcss'),
Cssnano({
preset: 'default',
}),
],
},
},
},
],
},
],
},
plugins: [
new MiniCssExtractPlugin({
filename: 'styles.css',
}),
new PurgeCSSPlugin({
paths: [
// Add the paths to your template files here
'./src//*.html',
'./src//.vue',
'./src/**/.jsx',
],
}),
],
};
This configuration sets up Webpack to process your CSS files with Tailwind CSS, PurgeCSS, and CSSnano.
Gulp
Gulp is a task runner and build system that can also be used to minify Tailwind CSS. To get started, first, install Gulp and the required plugins:
npm install gulp gulp-postcss gulp-purgecss cssnano tailwindcss
# or
yarn add gulp gulp-postcss gulp-purgecss cssnano tailwindcss
Next, create a gulpfile.js
in your project root directory and add the following configuration:
javascript
const gulp = require('gulp');
const postcss = require('gulp-postcss');
const purgecss = require('gulp-purgecss');
const cssnano = require('cssnano');
const tailwindcss = require('tailwindcss');
gulp.task('css', () => {
return gulp
.src('./src/css/*.css')
.pipe(postcss([tailwindcss(), cssnano()]))
.pipe(
purgecss({
content: [
// Add the paths to your template files here
'./src/**/*.html',
'./src/**/*.vue',
'./src/**/*.jsx',
],
}),
)
.pipe(gulp.dest('./dist/css'));
});
gulp.task('default', gulp.series('css'));
This Gulp configuration will process your CSS files with Tailwind CSS, PurgeCSS, and CSSnano when you run the gulp
command.
PostCSS
PostCSS is a CSS post-processor that can be used to minify Tailwind CSS as well. First, install PostCSS and the required plugins:
npm install postcss postcss-cli purgecss cssnano tailwindcss
# or
yarn add postcss postcss-cli purgecss cssnano tailwindcss
Create a postcss.config.js
file in your project root directory and add the following configuration:
javascript
const cssnano = require('cssnano');
const tailwindcss = require('tailwindcss');
const purgecss = require('@fullhuman/postcss-purgecss')({
content: [
// Add the paths to your template files here
'./src/**/*.html',
'./src/**/*.vue',
'./src/**/*.jsx',
],
defaultExtractor: (content) => content.match(/[\\w-/:]+(?<!:)/g) || [],
});
module.exports = {
plugins: [
tailwindcss(),
...(process.env.NODE_ENV === 'production' ? [purgecss, cssnano()] : []),
],
};
Now, you can use the postcss
command to process your CSS files with Tailwind CSS, PurgeCSS, and CSSnano:
npx postcss src/css/*.css -o dist/css/styles.css
Verifying Minification
After using one of the methods above to minify your Tailwind CSS, it's essential to verify that your CSS files have been minified correctly. You can do this by checking the file size of your output CSS files and comparing them to the original, unminified files.
Another way to verify minification is to open the minified CSS file and ensure that it is a single line of code with no unnecessary whitespace or comments.
Best Practices for Production Readiness
- Version control: Use a version control system like Git to track your changes and maintain a clean codebase. This allows you to revert to a previous version of your code if something goes wrong during the minification process.
- Testing: Always test your website after minifying your CSS to ensure that everything is working as expected. Check for any broken styles or functionality, and fix any issues before deploying to production.
- Continuous integration: Implement a continuous integration (CI) pipeline to automate the build process, including minification. This helps maintain a consistent production environment and reduces the risk of errors during deployment.
- Documentation: Keep your project documentation up-to-date, including instructions on how to minify your CSS and any configuration changes made to your build tools.
By following these best practices, you can maintain a production-ready codebase and ensure your website's performance remains optimal.
Conclusion
Minifying Tailwind CSS is crucial for optimizing your website's performance and ensuring fast page load times. This article has covered various techniques and tools to minify Tailwind CSS for production readiness, including using PurgeCSS, CSSnano, Webpack, Gulp, and PostCSS. Choose the method that best fits your project's requirements and workflow, and always follow best practices for maintaining a production-ready codebase.
Frequently Asked Questions
How do I update my Tailwind CSS configuration when minifying for production?
You don't need to modify your Tailwind CSS configuration file to minify it for production. The minification process is handled by external tools like PurgeCSS, CSSnano, Webpack, Gulp, or PostCSS. Just follow the steps outlined in this article to minify your Tailwind CSS using one of these tools.
Is it necessary to minify my CSS if I'm already using PurgeCSS to remove unused styles?
Yes, minifying your CSS is still important even if you're using PurgeCSS. While PurgeCSS removes unused styles, minification further optimizes your CSS by compressing the remaining styles, eliminating unnecessary whitespace, and removing comments. This process helps reduce file size and improve website performance.
Can I use other CSS minifiers instead of CSSnano?
A: Yes, you can use other CSS minifiers instead of CSSnano. Some popular alternatives include clean-css and uglifycss. Just make sure to follow the respective installation and configuration instructions for the chosen minifier in conjunction with Tailwind CSS.
Can I use these minification techniques with other CSS frameworks?
A: Yes, the techniques described in this article for minifying Tailwind CSS can be applied to other CSS frameworks or custom CSS files as well. Just ensure you adjust the configuration settings and paths to match the requirements of your specific framework or project.
Will minifying my CSS affect the debugging process during development?
A: Minifying your CSS can make it difficult to debug your styles during development. To avoid this issue, only minify your CSS when preparing your project for production deployment. You can do this by setting up your build tools to minify your CSS only in a production environment, as demonstrated in the Webpack and PostCSS examples in this article.