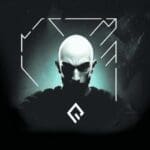
How To Handle Binary Requests In Node.js, Ruby on Rails, And Django
Introduction
Handling binary requests is an essential skill for modern web development, as binary data transfer is required for various operations like file uploads, image processing, or audio and video streaming. This article will discuss how to handle binary requests in three popular web frameworks: Node.js, Ruby on Rails, and Django.
Handling Binary Requests in Node.js
Using Buffers
In Node.js, binary data is represented using the Buffer
class, which allows developers to create and manipulate binary data efficiently. Buffers can be created from strings, arrays, or other data sources and can be manipulated using various methods provided by the Buffer
class.
Receiving Binary Data
To handle binary data in an Express.js application, developers can use the req.on('data', ...)
and req.on('end', ...)
events to read and process the incoming binary data:
const express = require('express');
const app = express();
app.post('/binary', (req, res) => {
const chunks = [];
req.on('data', (chunk) => {
chunks.push(chunk);
});
req.on('end', () => {
const binaryData = Buffer.concat(chunks);
console.log(binaryData);
res.sendStatus(200);
});
});
app.listen(3000, () => {
console.log('Server listening on port 3000');
});
Sending Binary Data
To send binary data in an Express.js application, developers should set the appropriate Content-Type
header and use the res.send()
or res.end()
methods:
const binaryData = Buffer.from('example binary data', 'binary');
res.setHeader('Content-Type', 'application/octet-stream');
res.send(binaryData);
Handling File Uploads
The multer
middleware can be used to handle file uploads in an Express.js application. Developers can configure file storage and retrieval options using the multer
configuration:
const multer = require('multer');
const upload = multer({ dest: 'uploads/' });
app.post('/upload', upload.single('file'), (req, res) => {
console.log('File uploaded:', req.file);
res.sendStatus(200);
});
Handling Binary Requests in Ruby on Rails
Using String Encodings
In Ruby, binary data can be represented using strings with the BINARY
or ASCII-8BIT
encoding:
binary_data = "example binary data".encode(Encoding::BINARY)
Receiving Binary Data
In a Ruby on Rails application, binary data can be accessed through the request.body
object. Developers can read and process binary data using the read
method:
def binary
binary_data = request.body.read
puts binary_data
head :ok
end
Sending Binary Data
To send binary data in a Ruby on Rails application, developers should set the appropriate Content-Type
header and use the send_data
or send_file
methods:
binary_data = "example binary data".encode(Encoding::BINARY)
send_data binary_data, type: 'application/octet-stream', disposition: 'inline'
Handling File Uploads
Ruby on Rails provides Active Storage for handling file uploads. Developers can configure file storage and retrieval options using Active Storage's configuration:
class UploadsController < ApplicationController
def create
@upload = Upload.create(file: params[:file])
redirect_to @upload
end
end
Handling Binary Requests in Django
Using Bytes and ByteArrays
In Python, binary data can be represented using the bytes
and bytearray
data types:
binary_data = b'example binary data'
Receiving Binary Data
In a Django application, binary data can be accessed through the request.body
attribute. Developers can handle binary data within Django views:
from django.http import HttpResponse
def binary(request):
binary_data = request.body
print(binary_data)
return HttpResponse(status=200)
Sending Binary Data
To send binary data in a Django application, developers should set the appropriate Content-Type
header and use the HttpResponse
or FileResponse
classes:
from django.http import HttpResponse
binary_data = b'example binary data'
response = HttpResponse(binary_data, content_type='application/octet-stream')
return response
Handling File Uploads
Django provides built-in support for handling file uploads. Developers can configure file storage and retrieval options using Django's file handling capabilities:
from django.core.files.storage import default_storage
def upload(request):
file = request.FILES['file']
default_storage.save(file.name, file)
return HttpResponse(status=200)
Best Practices for Handling Binary Data
Security Considerations
When handling binary data, developers should always validate and sanitize the data to protect against potential security risks. Additionally, developers should implement measures to protect against common file upload vulnerabilities, such as limiting file size, validating file types, and securing file storage.
Performance Optimization
Optimizing the handling of binary data can improve application performance. Techniques such as reducing binary data size, implementing caching, and using compression strategies can lead to faster response times and reduced server load.
Conclusion
Handling binary requests is an essential skill for modern web developers, as it enables seamless data transfer and manipulation in various formats. By understanding how to handle binary data in popular web frameworks like Node.js, Ruby on Rails, and Django, developers can create more versatile and efficient web applications.
Frequently Asked Questions
1. How can I convert binary data to a specific format?
Binary data can be converted to specific formats using built-in or third-party libraries, depending on the desired format. For example, you can use the toString()
method in Node.js to convert binary data to a string with a specific encoding.
2. How can I handle large binary files efficiently?
Large binary files can be handled efficiently by streaming the data in smaller chunks instead of loading the entire file into memory. This can be achieved using built-in streaming APIs in your web framework or programming language.
3. How can I handle binary data in WebSocket communication?
WebSocket libraries typically provide support for sending and receiving binary data as ArrayBuffer
or Blob
objects. Consult the documentation of your WebSocket library for specific instructions on handling binary data.
4. How can I store and retrieve binary data from a database?
Storing and retrieving binary data from a database can be achieved using Binary Large Object (BLOB) data types or equivalent data types provided by your database system. Consult the documentation of your database system for specific instructions on handling binary data.
5. What are the security risks associated with handling binary data?
Security risks associated with handling binary data include potential vulnerabilities related to file uploads, data validation, and data sanitization. Developers should always validate and sanitize binary data, limit file sizes, validate file types, and secure file storage to mitigate these risks.