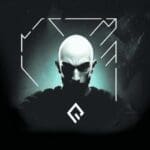
Handling Events In The DOM: How To Use addEventListener and removeEventListener
Introduction
When working with web development, one essential aspect is creating and managing interactivity between users and web applications. This interactivity is often driven by events in the Document Object Model (DOM). The DOM represents the structure of an HTML or XML document as a tree data structure, allowing developers to easily manipulate the content, structure, and style of a web page.
Understanding how to handle events in the DOM is crucial for building responsive, dynamic web applications. In this article, we'll explore the basics of events in JavaScript, such as how they are initiated and propagated, and dive into how to work with the addEventListener
and removeEventListener
methods to effectively manage events within web development projects.
Understanding DOM Events
DOM events are actions or occurrences that happen within the browser window as users interact with web pages. These events can be triggered by various user actions, such as clicking a button, hovering over an element, or even simply loading a page.
Some of the common types of events include:
- Click: Triggered when a user clicks on an element, such as a button or a link.
- Load: Triggered when the browser finishes loading a web page or a specific element.
- Mouseover: Triggered when a user moves the mouse cursor over an element.
- Mouseout: Triggered when a user moves the mouse cursor away from an element.
- Keydown: Triggered when a user presses a key on the keyboard.
- Submit: Triggered when a user submits a form.
When an event occurs, it goes through a process called event propagation, which involves two phases: capturing and bubbling. During the capturing phase, the event starts at the top of the DOM tree and propagates down towards the target element. Once the target element is reached, the bubbling phase begins, in which the event propagates upwards, back to the root of the DOM tree.
Understanding the event propagation process can help you effectively handle events and manipulate the DOM structure using JavaScript.
Introduction to addEventListener
The addEventListener
method is the most commonly used way to set up event listeners in JavaScript. It allows you to attach an event listener function or an object that implements the EventListener
interface to a specified DOM element. When the specified event occurs on the target element, the listener function is called, executing the provided code.
The addEventListener
method has three parameters:
- type: A string representing the type of event to listen for (e.g., "click", "load", "mouseover").
- listener: The function or object that defines the actions to be performed when the specified event occurs.
- options: An optional object that specifies characteristics of the event listener, such as whether it should listen during the capturing or bubbling phase, or if the listener should be removed after the first event occurrence.
Here's a practical example of using addEventListener
to attach a click event listener to a button element:
const button = document.getElementById('myButton');
button.addEventListener('click', function() {
alert('Button clicked!');
});
In this example, the addEventListener
method is called on a button element with the ID myButton
. When a user clicks the button, an alert will be displayed with the message "Button clicked!".
Working with Event Objects
When an event is triggered, the browser creates an event object containing information about the event, such as what type of event occurred, the target element that the event was triggered on, and other relevant properties. This event object is automatically passed to the event listener function as its first parameter.
Let's explore some of the most common properties of the event object:
- target: The DOM element that triggered the event. For example, if a user clicks a button, the button element would be the event target.
- currentTarget: The DOM element that the event listener is attached to. In most cases, this is the same as the target element.
- type: A string representing the type of event that occurred (e.g., "click", "load", "mouseover").
- preventDefault(): A method to prevent the default action associated with an event from occurring. For instance, if you want to handle form submission using AJAX, you can use this method to stop the browser from submitting the form and reloading the page.
- stopPropagation(): A method to stop the event from being propagated further in the event propagation process, either in the capturing or bubbling phase.
Here's a practical example illustrating how to use the event object to detect which mouse button was clicked:
const button = document.getElementById('myButton');
button.addEventListener('mousedown', function(event) {
if (event.button === 0) {
console.log('Left button clicked.');
} else if (event.button === 1) {
console.log('Middle button clicked.');
} else if (event.button === 2) {
console.log('Right button clicked.');
}
});
In this example, the mousedown
event listener is attached to a button element. The event object is used to determine which mouse button was clicked by checking its button
property and displaying the appropriate message in the console.
Removing Event Listeners
Knowing how to remove event listeners is just as important as attaching them. Failing to remove unneeded event listeners can cause memory leaks and performance issues in web applications. The removeEventListener
method allows you to detach an event listener that was previously registered with addEventListener
.
The removeEventListener
method accepts the same parameters as addEventListener
: the event type, the listener function, and the options object. However, to remove an event listener, you must provide the exact same function that was used to add the listener. This means you cannot use anonymous functions, as you cannot reference them later to remove the listener.
Here's an example demonstrating how to properly add and remove an event listener:
const button = document.getElementById('myButton');
function buttonClickHandler() {
alert('Button clicked!');
}
// Add the event listener
button.addEventListener('click', buttonClickHandler);
// Remove the event listener
button.removeEventListener('click', buttonClickHandler);
In this example, we first create a named function called buttonClickHandler
that displays an alert when the button is clicked. We then use this function to add a click event listener to the button element. Later, we can remove the event listener using the same function reference and the removeEventListener
method.
Event Delegation
Event delegation is a technique used to handle events efficiently, especially when working with a large number of similar DOM elements or dynamically added elements. Instead of attaching event listeners to each of these elements individually, event delegation leverages the event propagation process to handle events at a higher level in the DOM tree, typically on a common ancestor element.
The main benefits of event delegation include:
- Significantly improved performance and reduced memory usage, since fewer event listeners are attached and managed.
- Automatic handling of events for dynamically added elements that match the required criteria, without the need to attach listeners to new elements manually.
To implement event delegation, follow these steps:
- Attach the event listener to a common ancestor of the elements that require event handling.
- Use the
event.target
property to check if the target element matches the desired criteria (e.g., using thematches()
method or checking the element's class, tag, etc.). - If the target element matches the criteria, perform the necessary actions to handle the event.
Here's a practical example of event delegation with a list of items where each item can be marked as completed when clicked:
<ul id="toDoList">
<li class="item">Buy groceries</li>
<li class="item">Do the laundry</li>
<li class="item">Finish the project</li>
</ul>
const toDoList = document.getElementById('toDoList');
toDoList.addEventListener('click', function(event) {
// Check if the target element is an item in the list
if (event.target.matches('.item')) {
// Mark the item as completed by toggling the 'completed' class
event.target.classList.toggle('completed');
}
});
In this example, we attach a click event listener to the unordered list element. When a list item is clicked, the event bubbles up to the ancestor <ul>
element, and our listener function checks if the target element is a list item. If it is, the 'completed' class will be toggled on that list item.
Practical Application: Building an Interactive Web Page
Let's create a sample interactive web page using the knowledge gained from the previous sections. This web page will allow users to add items to a to-do list, mark items as completed, and remove items as needed.
Step 1: Create the HTML structure
Create the basic HTML structure for the web page, including a form for adding new items and an empty list container.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>To-Do List</title>
</head>
<body>
<h1>To-Do List</h1>
<form id="addItemForm">
<input type="text" id="itemInput" placeholder="Add a new item...">
<button type="submit">Add Item</button>
</form>
<ul id="toDoList"></ul>
</body>
</html>
Step 2: Attach event listeners
Using addEventListener
, attach the necessary event listeners to handle the form submission and the interaction with the to-do list items.
const addItemForm = document.getElementById('addItemForm');
const itemInput = document.getElementById('itemInput');
const toDoList = document.getElementById('toDoList');
// Event listener for form submission
addItemForm.addEventListener('submit', function(event) {
event.preventDefault();
const newItem = document.createElement('li');
newItem.textContent = itemInput.value;
newItem.classList.add('item');
toDoList.appendChild(newItem);
itemInput.value = '';
});
// Event listener for interacting with list items
toDoList.addEventListener('click', function(event) {
if (event.target.matches('.item')) {
event.target.classList.toggle('completed');
} else if (event.target.matches('.remove')) {
event.target.parentElement.remove();
}
});
In this example, we attached event listeners to handle the form submission and the interaction with the to-do list items. When users submit the form, a new item is added to the list. When users click on an item, it will be marked as completed with the 'completed' class.
Best Practices for Handling Events in the DOM
Having a solid understanding of how to handle events in the DOM is crucial for creating efficient and responsive web applications. Below are some best practices and general rules to keep in mind when working with events:
- Use event delegation: Utilize event delegation to improve performance and minimize memory usage, especially when dealing with a large number of similar elements or elements that are dynamically added to the DOM.
- Remove unneeded event listeners: Always remove event listeners when they are no longer needed to prevent memory leaks and performance issues. Remember to use the exact same function reference with the
removeEventListener()
method. - Avoid using inline event handlers: Although it's possible to use inline event handlers (e.g.,
<button onclick="myFunction()">
), this approach is generally discouraged due to reduced functionality, limited flexibility, and poor separation of concerns. Instead, useaddEventListener()
to attach event listeners. - Prevent default actions when necessary: Use the
preventDefault()
method to stop the browser's default behavior when you need to handle events differently, such as when submitting forms through AJAX.
Conclusion
Understanding and effectively handling events in the DOM is essential for modern web development. In this article, we've covered the basics of DOM events, the process of event propagation, and how to work with the addEventListener()
and removeEventListener()
methods to manage events.
We also discussed event delegation, a powerful technique that can significantly improve the performance of your web applications, especially when handling a large number of events. Finally, we covered best practices and general guidelines to follow when working with events in the DOM.
With this knowledge in mind, continue exploring and practicing the many ways to create interactive and dynamic web applications using JavaScript events.
Frequently Asked Questions
1. What is the difference between event.stopPropagation() and event.stopImmediatePropagation()?
While both methods are used to stop event propagation, they differ in scope of effect:
event.stopPropagation()
: Prevents the event from further propagating through the event propagation process (either in the capturing or bubbling phase), but it does not prevent other event listeners on the same element from being executed.event.stopImmediatePropagation()
: Not only stops the event propagation, but also prevents the execution of any other event listeners on the same element that were added after the current listener.
2. How can I attach the same event listener to multiple elements?
You can use a loop to iterate through a collection of elements and attach the event listener to each of them using addEventListener()
:
const buttons = document.querySelectorAll('.myButtons');
const handler = function() {
console.log('Button click');
};
buttons.forEach(button => {
button.addEventListener('click', handler);
});
3. What is the difference between event.target and event.currentTarget?
event.target
: Refers to the element that originally triggered the event. During the event propagation process, this reference does not change.event.currentTarget
: Refers to the element that the event listener is attached to. This reference changes during the event propagation process as the event propagates through different elements.
4. How can I determine which key was pressed during a keyboard event?
The event object contains a key
property that holds a string representation of the key that triggered the event. You can use this property within a handler for keyboard events such as keydown
or keyup
:
document.addEventListener('keydown', function(event) {
console.log('Key pressed:', event.key);
});
5. How do I attach an event listener that gets triggered only once?
You can use the options
parameter of the addEventListener()
method to specify that the event listener should only be executed once. Set the once
property to true
:
button.addEventListener('click', function() {
console.log('This will be executed only once.');
}, { once: true });
In this example, the event listener will be automatically removed after its first execution.