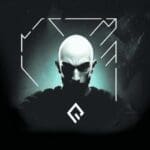
CSS Houdini - How To Create Custom CSS
Introduction
CSS Houdini empowers developers to extend the capabilities of CSS, providing more control over the look and feel of their web applications. This article will give you an in-depth understanding of CSS Houdini and teach you how to create custom CSS using its powerful APIs.
Overview of CSS Houdini
CSS Houdini is an umbrella term for a collection of technologies and APIs that enables developers to hook into the browser's rendering engine and create custom styling, layouts, and animations. It unlocks the "magic" of CSS and allows developers to push the boundaries of web design. Houdini addresses the limitations in CSS by giving us a direct link to the browser's rendering engine, thus extending the features and capabilities of CSS that were previously not possible.
The role of Houdini in extending the capabilities of CSS
CSS has always been a powerful tool for styling websites, but it sometimes falls short in certain aspects. This is where Houdini comes in, making it easier to create complex effects and customizations that were previously limited or not achievable through regular CSS. It offers a new, more flexible approach to web design, allowing developers to create custom features and properties that can be easily shared and used across projects. By harnessing the power of Houdini, developers can create more visually appealing and performant websites without relying on hacks or excessive JavaScript.
Understanding CSS Houdini
What is CSS Houdini?
CSS Houdini is a set of low-level APIs that allows developers to access and manipulate the browser's rendering engine directly. Essentially, the APIs serve as an extension to CSS, providing more control over styling, layout, and animations. The main purpose of Houdini is to make it easier for developers to create custom CSS properties, features, and enhancements to meet their project requirements.
The Houdini Task Force, a collaboration between major browser vendors such as Google, Mozilla, and Apple, is responsible for developing and standardizing the Houdini APIs. This task force aims to enhance the capabilities of CSS by making it easier for developers to extend the language and build advanced web applications, simplifying both the development process and the overall performance of the web.
Houdini APIs and Features
Houdini encompasses a variety of powerful APIs that developers can harness to create custom CSS properties, layouts, animations, and more. Some of the key APIs and features include:
- Properties and Values API: This API allows developers to define and register custom CSS properties, with specified types, initial values, and other property-specific rules that browsers can parse and understand.
- Layout API: With the Layout API, developers can create custom layout algorithms, defining how elements are sized, positioned, and rendered on the screen. This enables more advanced and intricate layouts than what traditional CSS offers.
- Paint API: The Paint API empowers developers to generate custom graphics, backgrounds, and visual effects directly in CSS, without having to rely on external images or SVG files.
- Animation Worklet API: This API facilitates creating custom animations and effects that can be triggered, controlled, and manipulated more efficiently by the browser's rendering engine, leading to better performance and smoother animations.
As CSS Houdini continues to evolve and grow, additional APIs will be introduced and existing ones will be refined, unlocking even more possibilities for web developers.
In the following sections, we will delve into each of these APIs and demonstrate how you can create custom CSS using Houdini's powerful features.
Creating Custom CSS with Houdini
Custom Properties and Values API
The Properties and Values API is the foundation of CSS Houdini, providing the ability to define and register custom CSS properties. These custom properties allow developers to create more complex and dynamic styles without the need for JavaScript or complex CSS tricks.
To create a custom CSS property, you first register the property using the CSS.registerProperty()
method. Here's an example of defining a custom property called --my-color
with a default value of red
and a specified syntax of a color:
CSS.registerProperty({
name: '--my-color',
syntax: '<color>',
initialValue: 'red',
inherits: false
});
In the above example, the syntax
field specifies the type of the property, which is a color value. The initialValue
field sets the default value to be red
, and the inherits
field determines whether the property inherits its value from its parent element (set to false
in this case).
After registering a custom property, you can use it in your CSS, just like any other CSS property:
.my-element {
background-color: var(--my-color);
}
With the Properties and Values API, you can create custom properties that suit your project, make your CSS more flexible, and improve code maintainability.
Custom Layout API
The Custom Layout API enables developers to create their own layout algorithms for more advanced and complex web designs. This API gives developers direct access to the browser's layout engine, allowing for the creation of custom layout logic that isn't achievable with standard CSS.
To implement a custom layout using the Layout API, you need to define the layout logic using JavaScript and then apply the layout worklet to your CSS. Here's a high-level overview of how you can create a custom layout and apply it to a web page:
- Create a JavaScript file (e.g.,
my-layout.js
) that defines your custom layout:
class MyLayout {
// Define your custom layout logic here
}
// Register the custom layout worklet
registerLayout('my-layout', MyLayout);
- Load the layout worklet from your HTML file:
<script>
if ("layoutWorklet" in CSS) {
CSS.layoutWorklet.addModule('my-layout.js');
}
</script>
- Apply the custom layout to an element by setting the
layout()
value in the CSSdisplay
property:
.my-element {
display: layout(my-layout);
}
The custom layout is now applied to the .my-element
class, and the specified layout logic will be used to render and position the element on the page.
Implementing custom layouts using the Layout API requires understanding the intricacies of browser layout rendering and can be complex, but it provides limitless possibilities for designing unique and intricate layouts that go beyond the capabilities of traditional CSS.
Custom Paint API
The Custom Paint API allows developers to create custom graphics, backgrounds, visual effects, and much more, all within CSS. Using this API, you can generate dynamic visuals without relying on external images or SVG files, resulting in faster load times and easier management of design assets.
In order to create a custom painting using the Paint API, you must define your painting logic within a JavaScript file and then apply the paint worklet to your CSS. Here's a step-by-step guide on how to create and register a custom paint worklet:
- Define your custom painting logic in a JavaScript file (e.g.,
my-painting.js
):
class MyPainting {
paint(context, size) {
// Define your custom painting logic here
context.fillStyle = 'blue';
context.fillRect(0, 0, size.width, size.height);
}
}
// Register the custom paint worklet
registerPaint('my-painting', MyPainting);
In the example above, the paint()
method contains the painting logic for your custom paint worklet, and registerPaint()
is used to register it with the browser.
- Load the paint worklet in your HTML file:
<script>
if ('paintWorklet' in CSS) {
CSS.paintWorklet.addModule('my-painting.js');
}
</script>
- Apply the custom paint worklet to an element in your CSS:
.my-element {
background-image: paint(my-painting);
}
Now, the custom painting logic will be applied as the background for .my-element
. The Paint API gives developers creative freedom to design unique and dynamic visuals while keeping performance in mind.
Custom Animations with Animation Worklet API
The Animation Worklet API enables developers to build complex and performant animations that are more controllable and efficient compared to traditional CSS animations. This API provides direct access to the browser's rendering engine, resulting in better performance and smoother animations, especially when dealing with large or intricate designs.
To create a custom animation using the Animation Worklet API, follow these steps:
- Define your custom animation logic in a JavaScript file (e.g.,
my-animation.js
):
class MyAnimation {
animate(currentTime, effect) {
// Define your custom animation logic here
const progress = currentTime % 1000 / 1000; // Range: 0 to 1
effect.localTime = progress * effect.timeline.duration;
}
}
// Register the custom animation worklet
registerAnimator('my-animation', MyAnimation);
In the example above, the animate()
method contains the custom animation logic, and registerAnimator()
is used to register the worklet with the browser.
- Load the animation worklet in your HTML file:
<script>
if ('animationWorklet' in CSS) {
CSS.animationWorklet.addModule('my-animation.js');
}
</script>
- Apply the custom animation worklet to an element in your CSS:
.my-element {
animation: my-animation 1s infinite;
animation-worklet: my-animation;
}
The custom animation logic is now applied to .my-element
. The Animation Worklet API allows developers to create intricate and responsive animations while maintaining performance and great user experiences.
With these powerful APIs at your disposal, CSS Houdini empowers you to create custom CSS properties, layouts, animations, and graphics that were once impossible or limited by traditional CSS. Your imagination and creativity now have more room to flourish.
Best Practices and Tips
When working with CSS Houdini to create custom CSS properties, layouts, animations, or graphics, it's important to keep in mind some best practices and tips to ensure your creations are accessible, maintainable, and performant.
Performance Considerations
While Houdini brings many new possibilities and capabilities, it's important to consider the performance impact of your custom CSS. The following tips can help you ensure that your Houdini customizations are performant and optimized:
- Avoid unnecessary work: When implementing custom properties, layouts, animations, or graphics, make sure that any computation or rendering is only done when necessary. For example, avoid creating overly complex paint worklets that could slow down your page rendering.
- Optimize your code: Maintain clean and efficient code when implementing Houdini APIs. Use efficient algorithms, minimize unnecessary computations, and leverage browser optimizations whenever possible.
- Measure performance: Always test and measure the performance of your custom Houdini CSS. Utilize browser developer tools, such as Google Chrome's DevTools, to monitor and profile your work, identifying any bottlenecks or areas that can be optimized.
Fallbacks and Progressive Enhancement
Not all browsers support CSS Houdini, which means that some users might encounter a degraded experience, or worse, a completely broken layout. To ensure your custom Houdini CSS works seamlessly across various browsers, follow these guidelines:
- Provide fallback styles: Make sure to include fallback styles for browsers that do not support CSS Houdini. These styles should serve as a baseline, ensuring that users with unsupported browsers still have a usable and accessible experience.
- Use feature detection: Check for Houdini API support in the user's browser using feature detection. This allows you to conditionally apply Houdini customizations only when the APIs are supported, ensuring that users on unsupported browsers receive the appropriate fallback styles.
- Embrace progressive enhancement: Apply the concept of progressive enhancement to your Houdini customizations. Build and design your site with a solid foundation, and then layer on Houdini features as enhancements for supported browsers. This approach ensures that your site is accessible and functional for all users, regardless of their browser or device.
Collaboration and Sharing
Houdini's true potential lies in its ability to enable developers to create reusable, shareable custom CSS. Consider the following recommendations to collaborate and contribute to the web development community:
- Share your custom properties and worklets: Make your custom Houdini CSS available to other developers through open-source repositories and articles. Sharing your work can have a meaningful impact on the broader web development community and foster innovation.
- Contribute to Houdini-related projects: Participate in the development and improvement of Houdini by contributing to open-source projects, reporting bugs, or submitting feature requests. By collaborating with other developers, you help advance the state of the art in web design and development.
- Stay up to date with Houdini advancements: As an evolving technology, CSS Houdini is always changing and introducing new APIs and features. Stay informed about the latest developments by following the work of the Houdini Task Force, participating in relevant web development forums, and engaging with the community.
Conclusion
CSS Houdini opens the door to a new level of web development, allowing developers to create custom CSS properties, layouts, animations, and graphics that go beyond the limitations of traditional CSS. By harnessing the power of Houdini's APIs, web developers can push the boundaries of web design and create more visually compelling, performant, and accessible websites.
As CSS Houdini continues to evolve and grow, it's important to stay up-to-date with the latest developments, best practices, and tips. Embrace the exciting world of Houdini, and unlock the true potential of CSS in your projects.
The future of CSS Houdini is bright, with continuous development and standardization efforts by the Houdini Task Force and major browser vendors. By adopting Houdini, web developers become a part of this innovative movement, helping shape the future of web design and development. Embrace the potential of CSS Houdini to create extraordinary web experiences that inspire and captivate users around the globe.
Frequently Asked Questions
What browsers currently support CSS Houdini?
The level of CSS Houdini support varies among browsers. Some Houdini APIs are already supported in modern browsers like Chrome, Edge, and Opera, while others are still under development or only available through experimental flags in these browsers. Firefox and Safari are more limited in their current Houdini support. To check the current status of Houdini API support in various browsers, consult resources like Can I use or MDN Web Docs.
Is CSS Houdini suitable for production websites?
Given the varying levels of support across browsers, it's essential to implement fallbacks and progressive enhancement techniques when using CSS Houdini on production websites. You can create unique and innovative web experiences using Houdini, but always ensure that your site remains functional and accessible for users on unsupported browsers by providing fallback styles.
Can I use CSS Houdini with CSS preprocessors like Sass or LESS?
Yes, you can use CSS Houdini alongside CSS preprocessors like Sass or LESS. The custom properties and functions defined by Houdini can be integrated seamlessly with your existing preprocessor code. For example, the custom CSS properties registered with Houdini can be used as variables in your Sass or LESS files.
How does CSS Houdini affect website performance?
CSS Houdini can potentially improve website performance by offloading complex visual effects, animations, or layouts to the browser's rendering engine instead of using JavaScript or other workarounds. However, it's crucial to follow best practices, optimize your code, and avoid overloading your site with unnecessary computational work or complex rendering tasks.
Where can I learn more about CSS Houdini?
The best resources for learning more about CSS Houdini include the W3C's Houdini drafts, which contain detailed information about each API, as well as the Houdini Task Force GitHub repository, where you can find the latest updates and development progress. Additionally, various articles, tutorials, and talks are available from web developers experienced in working with CSS Houdini. These resources can provide valuable guidance, practical examples, and insightful information about using Houdini effectively in your web projects.