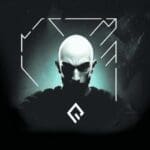
CSS Grid: Why Grid-Based Layouts Should Be In Every Design
Introduction
The CSS Grid Layout module has taken the web development world by storm, providing developers with powerful tools for creating complex and flexible grid-based layouts. In the rapidly evolving world of web design, grid-based layouts have become incredibly important for creating visually engaging and organized user interfaces.
In this article, we will explore the advantages of CSS Grid, discuss popular use cases, share best practices, and explain why grid-based layouts should be a part of every developer's toolkit.
Advantages of CSS Grid
CSS Grid offers a wide range of benefits compared to traditional layout systems. Let's explore some of the core advantages that make it a game-changer in modern web development.
Two-Dimensional Layout Control
A key feature of CSS Grid is its ability to control both rows and columns in a layout. By providing two-dimensional control, developers have much more flexibility in designing and structuring content. This stands in contrast to other layout systems like Flexbox, which mainly focuses on one-dimensional alignment (either in rows or columns).
Take the example below, which demonstrates how to create a two-dimensional grid layout using CSS Grid:
.container {
display: grid;
grid-template-columns: repeat(3, 1fr);
grid-template-rows: repeat(2, auto);
}
In this example, the container element is set as a grid with three equal columns and two rows with automatic height.
Simplified Markup
One of the key advantages of CSS Grid is its ability to reduce the need for additional wrappers and nested elements in HTML markup. With CSS Grid, developers can easily define the layout structure without resorting to convoluted and hard-to-maintain markup.
Consider the following example of a common layout with a header, sidebar, main content, and footer:
<div class="wrapper">
<header class="header">Header</header>
<aside class="sidebar">Sidebar</aside>
<main class="main">Main Content</main>
<footer class="footer">Footer</footer>
</div>
Using CSS Grid, we can easily position these elements without nesting or additional wrappers:
.wrapper {
display: grid;
grid-template-columns: 1fr 3fr;
grid-template-rows: auto auto auto;
grid-template-areas:
"header header"
"sidebar main"
"footer footer";
}
.header {
grid-area: header;
}
.sidebar {
grid-area: sidebar;
}
.main {
grid-area: main;
}
.footer {
grid-area: footer;
}
This simple markup and styling showcase the elegance and maintainability of using CSS Grid for layout management.
Responsive Design
In modern web design, responsiveness is crucial as users access content from a variety of devices and screen sizes. CSS Grid comes with built-in responsiveness, allowing developers to easily create fluid layouts that adapt to different viewports.
For instance, CSS Grid provides the fr
unit which represents a fraction of the available space in the grid container. This unit can be combined with other units like percentage, pixels, and ems to create responsive layouts.
To demonstrate, let's create a responsive layout that adjusts its columns based on the screen width:
.container {
display: grid;
grid-template-columns: repeat(auto-fit, minmax(200px, 1fr));
gap: 1rem;
}
In this example, we use the repeat()
, auto-fit
, and minmax()
functions to create a responsive grid layout that adjusts the number of columns based on the available space, with a minimum width of 200 pixels for each column. This responsiveness leads to a seamless experience for users across various devices and screen sizes.
Use Cases for CSS Grid
There are numerous practical use cases for CSS Grid, empowering developers to create a wide range of layouts and designs with ease. Let's explore some popular use cases that demonstrate the versatility of CSS Grid.
Page Layouts
One of the most common uses for CSS Grid is creating page layouts, where you can structure the various elements on a web page in a systematic and visually appealing manner. With CSS Grid, you can easily design page layouts with headers, footers, sidebars, and content areas.
For instance, let's create a simple, yet flexible page layout using CSS Grid:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>CSS Grid Page Layout</title>
<style>
body {
margin: 0;
}
.wrapper {
display: grid;
grid-template-columns: 1fr 3fr 1fr;
grid-template-rows: auto 1fr auto;
gap: 1rem;
height: 100vh;
}
.header {
grid-column: 1 / -1;
background-color: lightblue;
}
.sidebar {
background-color: lightgray;
}
.main {
background-color: #f0f0f0;
}
.footer {
grid-column: 1 / -1;
background-color: lightblue;
}
</style>
</head>
<body>
<div class="wrapper">
<header class="header">Header</header>
<aside class="sidebar">Sidebar</aside>
<main class="main">Main Content</main>
<footer class="footer">Footer</footer>
</div>
</body>
</html>
This example demonstrates how CSS Grid can be used to create a responsive and modern page layout with ease.
Responsive Galleries
Another powerful use case for CSS Grid is building responsive image galleries. Thanks to the built-in responsiveness and flexible sizing options offered by CSS Grid, creating visually engaging galleries that adjust to different screen sizes is simple and straightforward.
To illustrate this, let's create a responsive image gallery using CSS Grid:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>CSS Grid Gallery</title>
<style>
.gallery {
display: grid;
gap: 1rem;
grid-template-columns: repeat(auto-fill, minmax(200px, 1fr));
}
.gallery-item {
overflow: hidden;
}
img {
display: block;
width: 100%;
height: auto;
object-fit: cover;
transition: transform .2s ease-in-out;
}
.gallery-item:hover img {
transform: scale(1.1);
}
</style>
</head>
<body>
<div class="gallery">
<div class="gallery-item">
<img src="image1.jpg" alt="Image 1">
</div>
<div class="gallery-item">
<img src="image2.jpg" alt="Image 2">
</div>
<!-- Add more gallery items -->
</div>
</body>
</html>
In this example, we use CSS Grid to create a responsive image gallery that adjusts the number of columns based on the available screen width. On hover, the images zoom in slightly to provide visual feedback for the user.
These examples highlight merely a fraction of the many use cases for CSS Grid, which enable developers to create highly customizable and responsive layouts for web projects.
CSS Grid Best Practices
Adopting CSS Grid in your web projects not only involves understanding its core advantages and use cases but also implementing best practices to optimize your designs. Let's discuss some key best practices for working with CSS Grid.
Fallbacks for Older Browsers
While CSS Grid is widely supported by modern browsers, it's essential to provide fallbacks for older browsers that do not fully support this powerful layout module. One approach is to use feature queries and progressive enhancement techniques to ensure your design is usable across different browsers.
Consider the following example, where we provide a Flexbox-based fallback for browsers without CSS Grid support:
.container {
display: flex;
flex-wrap: wrap;
}
.item {
flex-grow: 1;
min-width: 200px;
}
@supports (display: grid) {
.container {
display: grid;
grid-template-columns: repeat(auto-fit, minmax(200px, 1fr));
gap: 1rem;
}
.item {
min-width: initial;
}
}
In this example, we define a Flexbox-based layout by default and then use the @supports
rule to apply CSS Grid-specific styles for browsers that support the Grid layout module.
Accessibility Considerations
Creating accessible web experiences should always be a priority, and this holds true when working with CSS Grid as well. Keep in mind that the visual order of elements in a grid might not match the source order in your HTML, which can lead to confusion for screen reader users and users who navigate using the keyboard.
To ensure proper focus order and screen reader support, make sure to test your grid-based layouts with various assistive technologies. Where necessary, provide accessible alternatives using techniques like ARIA roles and attributes, which will enhance the experience for all users.
Performance Optimization
Optimizing the performance of your grid-based layouts is crucial for providing a smooth and fast-loading user experience. Here are a few tips to enhance the performance of your CSS Grid designs:
- Minimize layout shifts: Avoid sudden jumps or shifts in the layout by defining the sizes of your grid tracks and items before the browser starts rendering the content.
- Use the most efficient sizing function: When using the
auto
keyword for sizing, keep in mind that it resolves to different values depending on the property it is used for. In some cases, this can lead to less efficient layout calculations. Instead, consider using more specific sizing functions likefr
,minmax()
, orfit-content()
to optimize performance. - Optimize images: For image-heavy grid layouts like galleries, ensure that you optimize images for web use to reduce load times and save bandwidth.
Conclusion
CSS Grid has revolutionized the way we approach layout design in web development. Its advantages, ranging from two-dimensional layout control to simplified markup and responsive design, make grid-based layouts an essential tool for any web developer.
As we've explored various use cases and best practices, it's clear that incorporating CSS Grid into your layouts enables you to craft visually engaging and organized user interfaces that adapt to different devices and screen sizes.
With CSS Grid at your disposal, building modern, responsive, and accessible web designs has never been more efficient and enjoyable.
Frequently Asked Questions
In this section, we'll answer some common questions related to CSS Grid and grid-based layouts.
What is the browser support for CSS Grid?
CSS Grid is widely supported by modern browsers, including the latest versions of Chrome, Firefox, Safari, Edge, and Opera. However, it's essential to provide fallbacks for older browsers that may not fully support CSS Grid.
To check the current browser support, you can visit Can I use, which provides up-to-date information on CSS Grid support and compatibility.
Can I use CSS Grid and Flexbox together?
Absolutely! CSS Grid and Flexbox can be used together to create complex and adaptable layouts. While CSS Grid is excellent for two-dimensional control over rows and columns, Flexbox excels in one-dimensional alignment and distribution of items.
By combining their strengths, you can achieve a wide variety of layout patterns that cater to different design needs. For instance, you can use CSS Grid for overall page layout and Flexbox for organizing content within grid items.
How do I create a gap between grid items?
Adding gaps between grid items is simple using the gap
property (or its individual row-gap
and column-gap
properties). The gap
property specifies the size of the space between rows and columns in a grid layout.
For example, to create a gap of 1rem between grid items, you can use the following CSS:
.container {
display: grid;
grid-template-columns: repeat(3, 1fr);
gap: 1rem;
}
How can I overlap items in a CSS Grid layout?
Overlapping items in a CSS Grid layout can be achieved by explicitly placing two or more items in the same grid area or by using negative values for the grid-row
or grid-column
property.
<div class="container">
<div class="item item1">1</div>
<div class="item item2">2</div>
</div>
.container {
display: grid;
grid-template-columns: repeat(2, 1fr);
grid-template-rows: repeat(2, auto);
}
.item1 {
grid-column: 1 / 3;
grid-row: 1;
}
.item2 {
grid-column: 2;
grid-row: 1;
}
In this example, the .item1
and .item2
elements share the same grid row, creating an overlap effect.
Can you nest CSS Grids?
Yes, you can nest CSS Grids by creating a grid container inside another grid container. This allows you to create more intricate and sophisticated layouts with various levels of nested grids.
For instance, let's create a nested grid structure:
<div class="wrapper">
<header class="header">Header</header>
<aside class="sidebar">Sidebar</aside>
<main class="main">
<div class="nested-grid">
<div class="nested-item">Item 1</div>
<div class="nested-item">Item 2</div>
</div>
</main>
<footer class="footer">Footer</footer>
</div>
.wrapper {
display: grid;
grid-template-columns: 1fr 3fr;
grid-template-rows: auto auto auto;
grid-template-areas:
"header header"
"sidebar main"
"footer footer";
}
.header {
grid-area: header;
}
.sidebar {
grid-area: sidebar;
}
.main {
grid-area: main;
}
.footer {
grid-area: footer;
}
.nested-grid {
display: grid;
grid-template-columns: repeat(2, 1fr);
gap: 1rem;
}
In this example, the .nested-grid
element is a grid container nested inside the .main
element. This allows for the creation of more complex and multi-dimensional layouts.