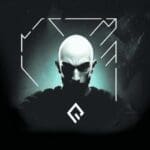
Building Reusable, Scalable Web Components From Scratch: Step-by-Step Guide
Introduction
Web components have revolutionized the way we develop modern web applications, providing us with a powerful tool to create reusable, scalable, and maintainable UI components. They allow developers to encapsulate markup, styles, and functionality into a single custom element, making it easier to share, reuse, and manage components across different projects and teams.
In this step-by-step guide, we will explore the core concepts of web components, including custom elements, shadow DOM, and HTML templates. We will then walk you through the process of building a scalable web component from scratch, testing it, integrating it into your applications, and optimizing its performance.
By the end of this guide, you will have a thorough understanding of the web components ecosystem and will be equipped with the knowledge to create your own reusable, scalable web components.
Understanding Web Components
Web components are a set of web platform APIs that allow you to create new custom, reusable, and encapsulated HTML tags for use in web pages and applications. There are three main technologies that make up web components: custom elements, shadow DOM, and HTML templates.
Custom Elements
Custom elements are the foundation of web components, allowing developers to create new HTML tags with custom behavior and properties. These new tags can be used alongside standard HTML tags and are treated as first-class citizens in the DOM.
To create a custom element, you need to define a new class that extends the HTMLElement
base class and register it with the customElements.define()
method. This method takes two arguments: the name of the custom element (which must include a hyphen) and the custom element class.
class MyCustomElement extends HTMLElement {
constructor() {
super();
// Initialize your custom element here
}
}
customElements.define('my-custom-element', MyCustomElement);
Custom elements have several lifecycle callbacks that can be used to manage their state and react to changes:
constructor
: Called when the custom element is created.connectedCallback
: Called when the custom element is added to the DOM.disconnectedCallback
: Called when the custom element is removed from the DOM.attributeChangedCallback
: Called when one of the custom element's attributes is added, changed, or removed.
Shadow DOM
Shadow DOM is a powerful feature that enables encapsulation of styles and markup within a custom element. This means that the internal structure of a web component is isolated from the rest of the DOM, preventing styles from leaking in or out and ensuring that the component's markup is not accidentally modified by external scripts.
To create a shadow DOM for a custom element, you need to call the attachShadow()
method in the element's constructor, passing an object with a mode
property set to either 'open'
or 'closed'
. This will create a shadow root, which is a special type of DOM node that hosts the shadow DOM.
class MyShadowElement extends HTMLElement {
constructor() {
super();
this.attachShadow({ mode: 'open' });
// Add your shadow DOM content here
}
}
With the shadow root in place, you can add content to the shadow DOM using standard DOM manipulation techniques, or by using the innerHTML
property.
HTML Templates
HTML templates provide a way to define reusable chunks of markup that can be cloned and inserted into the DOM as needed. To create an HTML template, you can use the <template>
tag, which is a special type of element that is not rendered by default.
<template id="my-template">
<div class="my-component">
<!-- Your component markup goes here -->
</div>
</template>
To use an HTML template in a web component, you can access the template element using querySelector()
, and then clone its content using the importNode()
method. The cloned content can then be appended to the shadow DOM or another part of the component.
class MyTemplatedElement extends HTMLElement {
constructor() {
super();
const shadowRoot = this.attachShadow({ mode: 'open' });
const template = document.querySelector('#my-template');
const content = document.importNode(template.content, true);
shadowRoot.appendChild(content);
}
}
With these three technologies combined, you can create powerful, reusable, and scalable web components that will make your web applications more maintainable and efficient. In the next section, we will dive into the process of creating a scalable web component from scratch.
Creating a Scalable Web Component
Creating a scalable web component involves designing the component, developing its custom element class, adding shadow DOM and templating, implementing its functionality, and testing it thoroughly. In this section, we'll walk you through each of these steps.
Designing the Component
Before you start writing code, it's important to plan out your web component's design by identifying its requirements and use cases. This will help you determine which properties, attributes, and methods the component should have, as well as how it should be structured and styled.
Consider the following questions when designing your component:
- What is the primary purpose of the component? What problem does it solve?
- What properties and attributes should the component have? How should users be able to configure it?
- What methods should the component expose for interacting with it programmatically?
- What events should the component emit, if any, to notify parent components or other parts of the application of changes or actions?
- How should the component be styled? Are there any theming options or variations that need to be supported?
Once you have a clear understanding of the component's requirements and use cases, you can move on to developing the custom element class.
Developing the Component
Creating the Custom Element Class
The first step in developing a web component is creating its custom element class. This class should extend the HTMLElement
base class and define a constructor that initializes the component's state and configuration.
Here's a basic example of a custom element class:
class MyComponent extends HTMLElement {
constructor() {
super();
// Initialize your component's state and configuration here
}
}
Next, you'll need to implement the custom element's lifecycle callbacks and attribute change handling. This will allow your component to react to changes in its attributes, as well as being added or removed from the DOM.
For example, if your component has a data-value
attribute that should be kept in sync with a property, you can implement the attributeChangedCallback
method like this:
class MyComponent extends HTMLElement {
static get observedAttributes() {
return ['data-value'];
}
attributeChangedCallback(name, oldValue, newValue) {
if (name === 'data-value') {
this.value = newValue;
}
}
}
Remember to register your custom element class using the customElements.define()
method:
customElements.define('my-component', MyComponent);
Adding Shadow DOM and Templating
Once your custom element class is set up, you can add a shadow DOM and an HTML template to encapsulate your component's markup and styles.
First, create an HTML template using the <template>
tag:
<template id="my-component-template">
<style>
/* Your component's CSS styles go here */
</style>
<div class="my-component">
<!-- Your component's markup goes here -->
</div>
</template>
Next, attach a shadow root to your custom element and import the template's content:
class MyComponent extends HTMLElement {
constructor() {
super();
const shadowRoot = this.attachShadow({ mode: 'open' });
const template = document.querySelector('#my-component-template');
const content = document.importNode(template.content, true);
shadowRoot.appendChild(content);
}
}
This will ensure that your component's styles are encapsulated and its markup is isolated from the rest of the DOM.
Adding Functionality
With the custom element class and shadow DOM in place, you can now add functionality to your web component by defining its methods and handling events.
For example, if your component needs to have a setValue()
method that updates its data-value
attribute and emits a value-changed
event, you can implement it like this:
class MyComponent extends HTMLElement {
setValue(value) {
this.setAttribute('data-value', value);
this.dispatchEvent(new CustomEvent('value-changed', { detail: value }));
}
}
Be sure to expose a clean API for your component's methods and properties, so that parent components or other parts of the application can interact with it easily and consistently.
Testing the Component
Finally, it's important to test your web component thoroughly to ensure that it works correctly and is compatible with different browsers. This can involve writing unit tests using frameworks like Jest or Mocha, as well as manual testing in various browser environments.
Here's an example of a simple unit test for a web component using Jest:
import { MyComponent } from './my-component';
describe('MyComponent', () => {
let component;
beforeEach(() => {
component = new MyComponent();
document.body.appendChild(component);
});
afterEach(() => {
document.body.removeChild(component);
});
test('should set data-value attribute when calling setValue()', () => {
component.setValue('test-value');
expect(component.getAttribute('data-value')).toBe('test-value');
});
test('should emit value-changed event when calling setValue()', () => {
const eventListener = jest.fn();
component.addEventListener('value-changed', eventListener);
component.setValue('test-value');
expect(eventListener).toHaveBeenCalledWith(expect.objectContaining({ detail: 'test-value' }));
});
});
Make sure to test your component's various states, configurations, and interactions to catch any potential issues or edge cases. Additionally, verify that your component works correctly in different browsers, including the latest versions of Chrome, Firefox, Safari, and Edge.
Integrating Web Components into Applications
Once your web component is fully developed and tested, you can integrate it into your applications by using it in HTML, JavaScript, or popular frontend frameworks like React, Vue, and Angular.
Using Web Components in HTML
To use your web component in an HTML file, simply include its custom element tag and set any desired attributes:
<my-component data-value="initial-value"></my-component>
The browser will automatically instantiate the custom element and render it according to its defined behavior and styles.
Using Web Components in JavaScript
If you need to create and configure your web component programmatically, you can use the document.createElement()
method:
const myComponent = document.createElement('my-component');
myComponent.setAttribute('data-value', 'initial-value');
document.body.appendChild(myComponent);
You can also manipulate your web component's properties and methods directly through JavaScript:
myComponent.setValue('new-value');
console.log(myComponent.value);
Using Web Components with Popular Frontend Frameworks
Web components can be integrated into modern frontend frameworks like React, Vue, and Angular with some additional setup and configuration.
For example, to use a web component in a React application, you might create a wrapper component that handles property and event binding:
import React, { useRef, useEffect } from 'react';
function MyComponentWrapper({ value, onValueChanged }) {
const ref = useRef();
useEffect(() => {
ref.current.setValue(value);
}, [value]);
useEffect(() => {
const listener = (event) => onValueChanged(event.detail);
ref.current.addEventListener('value-changed', listener);
return () => ref.current.removeEventListener('value-changed', listener);
}, [onValueChanged]);
return <my-component ref={ref}></my-component>;
}
Similar approaches can be taken for integrating web components into Vue and Angular applications. Be sure to consult the documentation for your specific framework for guidance on handling component communication and state management.
In the next section, we will explore how to optimize and distribute your web components for maximum performance and reusability.
Optimizing and Distributing Web Components
To ensure that your web components perform well and are easy to use in various projects, it's important to optimize their performance and package them for distribution. In this section, we'll discuss performance optimization techniques and best practices for publishing web components.
Performance Optimization
Web components can sometimes have a negative impact on application performance, particularly if they are large in size or have complex rendering logic. To mitigate these issues, consider the following optimization techniques:
- Lazy-loading: Instead of loading all your web components upfront, you can lazy-load them as needed using dynamic imports. This can significantly reduce the initial load time of your application and improve user experience.
// Lazy-loading a web component using dynamic import
async function loadMyComponent() {
await import('./my-component.js');
const myComponent = document.createElement('my-component');
document.body.appendChild(myComponent);
}
- Minimizing bundle size: Ensure that your web components are as small as possible by using minification and tree-shaking techniques. Tools like Webpack and Rollup can help you optimize your component bundles and remove any unused code.
- Optimizing rendering: To improve the rendering performance of your web components, consider using techniques like virtual DOM diffing, memoization, or offscreen rendering. These approaches can help reduce the amount of work required to update the DOM and keep your components running smoothly.
Publishing Web Components
Once your web components are optimized, you'll want to package them for distribution so that other developers can easily use them in their projects. The most common way to distribute web components is by publishing them to the npm registry.
To publish a web component to npm, follow these steps:
- Create a package.json file: In the root directory of your web component project, create a
package.json
file that contains metadata about your component, such as its name, version, and dependencies.
{
"name": "my-component",
"version": "1.0.0",
"main": "my-component.js",
"dependencies": {
// Your component's dependencies go here
}
}
- Build your component: Before publishing your web component, make sure to build it using a tool like Webpack or Rollup. This will generate a single, optimized JavaScript file that includes your component's code, styles, and any dependencies.
- Publish to npm: Once your component is built, you can publish it to npm by running the
npm publish
command in your project's root directory. You'll need to have an npm account and be logged in to the npm CLI to do this. - Document your component: To make it easy for other developers to use your web component, be sure to provide clear documentation that explains its purpose, usage, API, and any configuration options. This can be done in the form of a README file, a dedicated documentation website, or both.
With your web component published and documented, other developers can now easily install it using npm and import it into their projects.
Conclusion
Building reusable, scalable web components from scratch is a valuable skill that can greatly improve the maintainability and efficiency of your web applications. By following the step-by-step guide in this article, you should now have a solid understanding of the web components ecosystem and be well-equipped to create your own custom web components.
As you continue to develop your skills, remember to share your knowledge and creations with the development community. By contributing to the growing library of web components, you can help make the web a more powerful and versatile platform for everyone.
Frequently Asked Questions
1. Can I use web components with server-side rendering (SSR)?
Yes, web components can be used with server-side rendering, but there are some additional considerations to keep in mind. When using web components in an SSR context, you will need to ensure that your custom elements are defined on both the server and the client. Additionally, you may need to use a library like SkateJS or Hydrate to handle the rendering and hydration of your components.
2. How can I style a web component from outside its shadow DOM?
While the shadow DOM is designed to encapsulate a web component's styles, there are still ways to style a component from the outside. One approach is to use CSS custom properties (also known as CSS variables) to define themeable properties that can be overridden by the parent context. Another option is to expose a CSS part using the ::part()
pseudo-element, which allows external stylesheets to target specific elements within the shadow DOM.
3. How do I handle accessibility in web components?
Accessibility is an important concern when building web components, as it ensures that your components can be used by all users, including those with disabilities. To make your web components accessible, follow these best practices:
- Use semantic HTML elements and ARIA attributes to convey the component's structure and state.
- Ensure that your component is keyboard-navigable and focusable using the
tabindex
attribute. - Provide proper labels, descriptions, and alternative text for interactive elements and images.
- Test your component using accessibility tools like axe or Lighthouse.
4. Are web components supported in all browsers?
Web components are supported in modern browsers, including the latest versions of Chrome, Firefox, Safari, and Edge. However, older browsers like Internet Explorer 11 do not have native support for web components. If you need to support legacy browsers, you can use a set of polyfills called webcomponents.js to provide the necessary APIs.
5. How do I handle state management in web components?
State management in web components can be achieved through a combination of properties, attributes, events, and external data stores. For simple state management, you can use properties and attributes to store and synchronize the component's internal state, while emitting custom events to notify parent components or other parts of the application of state changes. For more complex state management, you can use external libraries like Redux or MobX to store and manage the application state outside of your components.