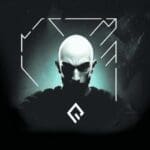
Authentication With Supabase: A Guide To Seamless User Management
Introduction
Supabase is an open-source alternative to Firebase, a powerful Backend-as-a-Service (BaaS) platform that aims to simplify the development of web and mobile applications. With its suite of tools and features, including authentication, real-time data synchronization, and serverless functions, Supabase is quickly becoming a popular choice for developers looking to create and manage user-friendly, scalable, and secure applications.
One of the key features of Supabase is its comprehensive authentication system. With just a few lines of code, developers can effortlessly implement user login, signup, password recovery, email verification, and social authentication. This enables developers to focus on building their application's core functionality, while Supabase handles the complexities of user management.
In this guide, we will explore how to set up and manage user authentication with Supabase. We'll cover everything from creating a new Supabase project and connecting it to your frontend application, to implementing various authentication methods and managing user data.
Setting Up Supabase
Creating a Supabase Project
To begin, you'll need to sign up for a Supabase account. Follow these steps to create a new project:
- Visit the Supabase website and click the "Start your project" button.
- Sign up using your preferred method (GitHub, Google, or email).
- Once logged in, click the "New Project" button on your dashboard.
- Enter a project name, select a region, and choose a database template. For this guide, you can use the "Blank Template" option.
- Click "Create new project."
Your Supabase project is now created, and you will be redirected to the project dashboard. Here, you can manage your database, authentication settings, and access your API keys.
Connecting to Your Supabase Project
To connect your frontend application to your Supabase project, you'll need to retrieve your project's API keys. Follow these steps:
- Navigate to the "API" section in your project dashboard.
- Copy the "URL" and "public" (anon) key values. These will be used to initialize the Supabase client in your frontend application.
Next, you'll need to integrate Supabase with your chosen frontend framework. For popular frameworks like React, Vue, and Angular, you can use the official Supabase libraries and plugins. Here are the installation steps for each:
React:
- Install the
@supabase/supabase-js
package:npm install @supabase/supabase-js
- Import and initialize the Supabase client:
import { createClient } from '@supabase/supabase-js'; const supabaseUrl = 'YOUR_SUPABASE_URL'; const supabaseAnonKey = 'YOUR_SUPABASE_ANON_KEY'; const supabase = createClient(supabaseUrl, supabaseAnonKey);
Vue:
- Install the
@supabase/supabase-js
package:npm install @supabase/supabase-js
- Import and initialize the Supabase client:
import { createClient } from '@supabase/supabase-js'; const supabaseUrl = 'YOUR_SUPABASE_URL'; const supabaseAnonKey = 'YOUR_SUPABASE_ANON_KEY'; const supabase = createClient(supabaseUrl, supabaseAnonKey);
Angular:
- Install the
@supabase/supabase-js
package:npm install @supabase/supabase-js
- Import and initialize the Supabase client in your
app.module.ts
file:import { createClient } from '@supabase/supabase-js'; const supabaseUrl = 'YOUR_SUPABASE_URL'; const supabaseAnonKey = 'YOUR_SUPABASE_ANON_KEY'; const supabase = createClient(supabaseUrl, supabaseAnonKey);
With your Supabase project connected to your frontend application, you can now begin implementing authentication and user management features.
Authentication in Supabase
Signup and Login
Supabase makes it easy to implement user signup and login functionalities in your application using the supabase.auth.signUp()
and supabase.auth.signIn()
methods. These methods handle user authentication and return a promise that resolves with the user's session data or an error.
To create a new user, call the supabase.auth.signUp()
method with the user's email and password:
async function signUp(email, password) {
try {
const { user, error } = await supabase.auth.signUp({ email, password });
if (error) {
// Handle error
console.error('Error during signup:', error.message);
} else {
// Handle successful signup
console.log('User signed up:', user);
}
} catch (error) {
// Handle unexpected errors
console.error('Unexpected error during signup:', error.message);
}
}
To authenticate an existing user, call the supabase.auth.signIn()
method with the user's email and password:
async function signIn(email, password) {
try {
const { user, error } = await supabase.auth.signIn({ email, password });
if (error) {
// Handle error
console.error('Error during sign in:', error.message);
} else {
// Handle successful sign in
console.log('User signed in:', user);
}
} catch (error) {
// Handle unexpected errors
console.error('Unexpected error during sign in:', error.message);
}
}
Remember to handle user input validation and error messages in your application. This ensures a smooth user experience and provides helpful feedback in case of any issues.
Social and Third-Party Authentication
Supabase also supports social and third-party authentication providers, such as Google, GitHub, GitLab, and more. This allows users to sign up and log in to your application using their existing accounts, making the process even more seamless and convenient.
To enable social authentication, follow these steps:
- Navigate to the "Authentication" section in your project dashboard.
- Select the "Settings" tab and scroll down to the "External OAuth Providers" section.
- Click "Enable" next to the provider you wish to use (e.g., Google, GitHub, GitLab).
- Follow the instructions to set up the provider and obtain the necessary credentials.
Once you have enabled a social authentication provider, you can implement the social login feature in your application using the supabase.auth.signIn()
method. Pass the provider's name as the provider
option:
async function signInWithProvider(provider) {
try {
const { user, error } = await supabase.auth.signIn({ provider });
if (error) {
// Handle error
console.error(`Error during sign in with ${provider}:`, error.message);
} else {
// Handle successful sign in
console.log(`User signed in with ${provider}:`, user);
}
} catch (error) {
// Handle unexpected errors
console.error(`Unexpected error during sign in with ${provider}:`, error.message);
}
}
For example, to authenticate a user using Google, call signInWithProvider('google')
.
Password Recovery
Supabase simplifies the process of password recovery by automating the generation and sending of password reset emails. To enable password recovery, follow these steps:
- Navigate to the "Authentication" section in your project dashboard.
- Select the "Settings" tab and scroll down to the "Emails" section.
- Toggle the "Enable password recovery" switch.
To send a password reset email to a user, call the supabase.auth.api.resetPasswordForEmail()
method with the user's email address:
async function sendPasswordResetEmail(email) {
try {
const { error } = await supabase.auth.api.resetPasswordForEmail(email);
if (error) {
// Handle error
console.error('Error sending password reset email:', error.message);
} else {
// Handle successful email sending
console.log('Password reset email sent');
}
} catch (error) {
// Handle unexpected errors
console.error('Unexpected error sending password reset email:', error.message);
}
}
You can also customize the password recovery email template from the "Emails" section in your project dashboard. This allows you to tailor the email's appearance and content to match your application's branding and messaging.
Email Verification
Email verification is an important feature to ensure that users provide valid email addresses and maintain the integrity of your application's user base. Supabase offers built-in email verification functionality that automatically sends a verification email to users upon registration.
To enable email verification, follow these steps:
- Navigate to the "Authentication" section in your project dashboard.
- Select the "Settings" tab and scroll down to the "Emails" section.
- Toggle the "Enable email verification" switch.
Once email verification is enabled, you can verify a user's email address by calling the supabase.auth.api.verifyUser()
method with the user's verification token. This token is included as a query parameter in the verification link sent to the user's email address.
async function verifyUserEmail(token) {
try {
const { error } = await supabase.auth.api.verifyUser(token);
if (error) {
// Handle error
console.error('Error verifying user email:', error.message);
} else {
// Handle successful email verification
console.log('User email verified');
}
} catch (error) {
// Handle unexpected errors
console.error('Unexpected error verifying user email:', error.message);
}
}
You can customize the email verification template from the "Emails" section in your project dashboard. This allows you to modify the email's appearance and content to align with your application's branding and messaging.
User Management
Retrieving User Information
Once a user is authenticated, you can access their information using the supabase.auth.user()
method. This method returns the currently logged-in user's session data, including their unique ID, email address, and additional metadata.
function getCurrentUser() {
const user = supabase.auth.user();
if (user) {
console.log('Current user:', user);
} else {
console.log('No user is currently signed in');
}
}
To fetch user data from the Supabase table, use the supabase.from()
method. This allows you to query the user's associated records in your database. For example, to fetch a user's profile data, you might do the following:
async function getUserProfile(userId) {
try {
const { data, error } = await supabase
.from('profiles')
.select('*')
.eq('user_id', userId)
.single();
if (error) {
// Handle error
console.error('Error fetching user profile:', error.message);
} else {
// Handle successful data retrieval
console.log('User profile:', data);
}
} catch (error) {
// Handle unexpected errors
console.error('Unexpected error fetching user profile:', error.message);
}
}
Updating User Information
Supabase allows you to update a user's information, such as their email address, password, and metadata, using the supabase.auth.update()
method. This method accepts an object containing the properties you wish to update and returns a promise that resolves with the updated user data or an error.
To update a user's email address, call the supabase.auth.update()
method with the new email:
async function updateUserEmail(newEmail) {
try {
const { user, error } = await supabase.auth.update({ email: newEmail });
if (error) {
// Handle error
console.error('Error updating user email:', error.message);
} else {
// Handle successful email update
console.log('User email updated:', user);
}
} catch (error) {
// Handle unexpected errors
console.error('Unexpected error updating user email:', error.message);
}
}
Similarly, you can update a user's password or metadata by passing the new password or metadata object:
async function updateUserPassword(newPassword) {
// ...
const { user, error } = await supabase.auth.update({ password: newPassword });
// ...
}
async function updateUserMetadata(metadata) {
// ...
const { user, error } = await supabase.auth.update({ data: metadata });
// ...
}
User Roles and Access Control
Supabase offers a powerful role-based access control (RBAC) system to manage user permissions and secure your application's data. By default, every Supabase user is assigned the "authenticated" role, which grants them access to their own data.
To create custom roles and permissions, navigate to your project dashboard and follow these steps:
- Click on the "Authentication" section.
- Select the "Roles" tab.
- Click the "Create role" button to define a new role with its associated permissions.
You can assign custom roles to users by updating their role
property in the Supabase table:
async function updateUserRole(userId, newRole) {
try {
const { error } = await supabase
.from('users')
.update({ role: newRole })
.eq('id', userId);
if (error) {
// Handle error
console.error('Error updating user role:', error.message);
} else {
// Handle successful role update
console.log('User role updated');
}
} catch (error) {
// Handle unexpected errors
console.error('Unexpected error updating user role:', error.message);
}
}
Logging Out Users
To log out a user, call the supabase.auth.signOut()
method. This clears the user's session data and redirects them to the authentication provider's logout page, if applicable.
async function signOut() {
try {
const { error } = await supabase.auth.signOut();
if (error) {
// Handle error
console.error('Error during sign out:', error.message);
} else {
// Handle successful sign out
console.log('User signed out');
}
} catch (error) {
// Handle unexpected errors
console.error('Unexpected error during sign out:', error.message);
}
}
It's important to handle logout events and user state changes in your application. This ensures that your application's UI is updated accordingly, and any sensitive data is protected from unauthorized access.
Securing Your Application
Supabase provides various features to help you secure your application and protect user data. One such feature is Row Level Security (RLS), which allows you to define access control policies directly at the database level.
Using Supabase's Row Level Security (RLS) for Data Access Control
To enable RLS on a table and create access control policies, follow these steps:
- Navigate to the "Database" section in your project dashboard.
- Select the table you wish to secure.
- Click the "Settings" tab and toggle the "Enable Row Level Security" switch.
- Create your access control policies using SQL expressions. These expressions define the conditions under which a user can access, insert, update, or delete data in the table.
For example, you can create a policy that restricts access to a user's own data:
CREATE POLICY "Access own data" ON your_table
FOR SELECT USING (auth.role() = 'authenticated' AND auth.uid() = user_id);
By using RLS, you can ensure that users can only access and modify data they are authorized to interact with.
Implementing Server-Side Logic with Supabase Functions
Supabase Functions allow you to run server-side logic in response to certain events or API requests. This can be useful for validating user input, enforcing business rules, and performing complex data transformations.
To create a Supabase Function, follow these steps:
- Navigate to the "Functions" section in your project dashboard.
- Click the "Create function" button.
- Choose a trigger type (e.g., database trigger, scheduled event, or API endpoint).
- Configure your function settings, such as the function name, schedule, or event source.
- Write your function code using JavaScript or TypeScript.
For example, you might create a function that validates user input before inserting it into the database:
// Function triggered by an "INSERT" event on the "your_table" table
export default async function validateInput(event) {
const { new: newItem } = event.data;
// Validate the newItem object
if (isValid(newItem)) {
// If valid, insert the item into the database
return { data: newItem };
} else {
// If invalid, return an error
return { error: { message: 'Invalid input' } };
}
}
By implementing server-side logic with Supabase Functions, you can further enhance your application's security and maintain data integrity.
Conclusion
In this guide, we explored the various authentication and user management features offered by Supabase. From setting up a new project and connecting it to your frontend application, to implementing user signup, login, password recovery, and email verification, Supabase simplifies the process of managing users in your web application.
Furthermore, we covered how to retrieve and update user information, manage user roles, and log out users. We also discussed securing your application using Supabase's Row Level Security and server-side logic with Supabase Functions.
With this knowledge, you are well-equipped to integrate Supabase into your web applications and create seamless, secure, and user-friendly experiences. So go ahead and explore the potential of Supabase in your next project!
Frequently Asked Questions
1. How do I customize the appearance of Supabase authentication emails?
To customize the appearance of Supabase authentication emails, such as password recovery and email verification messages, follow these steps:
- Navigate to the "Authentication" section in your project dashboard.
- Select the "Settings" tab and scroll down to the "Emails" section.
- Click the "Edit template" button next to the email type you wish to customize.
- Modify the email's subject, HTML content, and plain text content as desired.
- Click "Save" to apply your changes.
2. Can I use Supabase with mobile applications?
Yes, you can use Supabase with mobile applications. The @supabase/supabase-js
library, which is used to interact with the Supabase API, can be integrated into mobile applications built with React Native, Ionic, or other popular mobile frameworks. Simply follow the same installation and setup process as you would for a web application.
3. Is it possible to implement multi-factor authentication (MFA) with Supabase?
As of now, Supabase does not have built-in support for multi-factor authentication (MFA). However, you can implement MFA in your application using third-party services or libraries, such as Authy or Google Authenticator.
4. How do I handle real-time updates in Supabase?
Supabase provides real-time data synchronization through its @supabase/realtime-js
library. To listen for real-time updates on a table, use the supabase.from()
method followed by the .on()
method:
supabase
.from('your_table')
.on('INSERT', (payload) => {
console.log('New row added:', payload.new);
})
.subscribe();
You can listen for various events, such as INSERT
, UPDATE
, DELETE
, or *
(all events), and update your application's state accordingly.
5. How do I migrate my existing user data to Supabase?
To migrate your existing user data to Supabase, you can follow these steps:
- Export your existing user data in a format compatible with Supabase, such as CSV or JSON.
- In your Supabase project dashboard, navigate to the "Database" section.
- Create a new table with the appropriate schema to match your user data.
- Click the "Import" button in the table view.
- Upload your exported user data file and configure the import settings.
- Click "Start import" to import your user data into Supabase.
Once your user data is imported, you can update your application's authentication and data access logic to use Supabase's authentication and storage features.