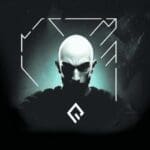
5 NoSQL Query Languages for Efficient Data Retrieval and Manipulation
Introduction
In recent years, NoSQL (Not Only SQL) databases have gained significant popularity due to their ability to handle large volumes of unstructured and semi-structured data, as well as their flexibility, scalability, and performance. Unlike traditional SQL databases, NoSQL databases do not rely on a fixed schema or relational model, allowing them to store and manage data in a variety of formats such as key-value, document, column-family, or graph-based data models.
Despite the differences between SQL and NoSQL databases, the process of retrieving, manipulating, and storing data remains a critical aspect of any database system. This is where query languages come into play. Query languages are essential tools that allow developers and data analysts to interact with the database and perform operations on the data stored within. In this article, we will explore five powerful NoSQL query languages that can help you effectively retrieve and manipulate data in various NoSQL databases.
1. MongoDB Query Language (MQL)
Overview and Features
MongoDB Query Language (MQL) is the native query language for MongoDB, a widely used NoSQL document-based database. MongoDB stores data in flexible, JSON-like documents called BSON (Binary JSON), which allows for a more expressive and dynamic schema design. MQL is designed to be simple and easy to use, offering developers a familiar JSON-based syntax for querying and manipulating data.
Key features of MQL include:
- JSON-like documents for a flexible and expressive schema design
- Support for ad-hoc queries, indexing, and real-time aggregation
- A rich set of query operators for filtering, projection, and updating data
- Horizontal scalability through sharding and automatic data distribution
Basic Operations
MQL supports a comprehensive set of CRUD (Create, Read, Update, Delete) operations for interacting with the data in a MongoDB database. Some common MQL operations include:
find()
: Retrieve documents from a collection that match a specified filter or criteriainsertOne()
: Insert a single document into a collectionupdateOne()
: Update a single document in a collection that matches a specified filterdeleteOne()
: Delete a single document from a collection that matches a specified filter
Here's an example of how to use these basic MQL operations:
// Find all documents in the "users" collection
db.users.find({});
// Insert a new document into the "users" collection
db.users.insertOne({
name: "Alice",
age: 30,
city: "New York"
});
// Update the age of the user named "Alice"
db.users.updateOne(
{ name: "Alice" },
{ $set: { age: 31 } }
);
// Delete a user with the name "Alice"
db.users.deleteOne({ name: "Alice" });
Advanced Operations
In addition to basic CRUD operations, MQL offers a powerful aggregation framework and a wide array of query operators for performing complex data processing tasks. Some examples of advanced MQL operations include:
$lookup
: Perform a left outer join between two collections on a specified field$group
: Group documents by a specified expression and apply accumulator functions to each group$unwind
: Deconstruct an array field from input documents to generate a document for each element
Here's an example demonstrating the use of advanced MQL operations:
// Find the total number of orders per customer and sort by total in descending order
db.orders.aggregate([
{ $lookup: {
from: "customers",
localField: "customerId",
foreignField: "_id",
as: "customerInfo"
}
},
{ $group: {
_id: "$customerId",
totalOrders: { $sum: 1 },
totalAmount: { $sum: "$amount" }
}
},
{ $unwind: "$customerInfo" },
{ $sort: { totalAmount: -1 } }
]);
2. Cassandra Query Language (CQL)
Overview and Features
Cassandra Query Language (CQL) is a query language designed for Apache Cassandra, a highly scalable and distributed NoSQL column-family database. CQL provides a SQL-like syntax for interacting with Cassandra, making it easier for developers familiar with SQL to work with Cassandra.
Key features of CQL include:
- A simple and familiar SQL-like syntax for querying and manipulating data
- Support for schema definition, data manipulation, and querying
- Distributed storage and high availability through partitioning and replication
- Tunable consistency levels for balancing data consistency and availability
Basic Operations
CQL supports a wide range of CRUD operations for managing data in a Cassandra database. Some common CQL operations include:
SELECT
: Retrieve rows from a table that match a specified filter or criteriaINSERT
: Insert a new row into a tableUPDATE
: Update the values of columns in rows that match a specified filterDELETE
: Delete rows from a table that match a specified filter
Here's an example of how to use these basic CQL operations:
-- Find all rows in the "users" table
SELECT * FROM users;
-- Insert a new row into the "users" table
INSERT INTO users (id, name, age, city) VALUES (1, 'Alice', 30, 'New York');
-- Update the age of the user with id 1
UPDATE users SET age = 31 WHERE id = 1;
-- Delete the row with id 1 from the "users" table
DELETE FROM users WHERE id = 1;
Advanced Operations
Cassandra offers several advanced features and operations for managing data distribution, consistency, and performance. Some examples of advanced CQL operations include:
CREATE INDEX
: Create an index on a column to enable efficient queryingCREATE MATERIALIZED VIEW
: Create a materialized view that automatically updates based on a specified base tableUSING TIMESTAMP
: Specify a custom timestamp for an operation to control the order of updates
Here's an example demonstrating the use of advanced CQL operations:
-- Create an index on the "city" column in the "users" table
CREATE INDEX users_city_idx ON users (city);
-- Create a materialized view of users grouped by city
CREATE MATERIALIZED VIEW users_by_city AS
SELECT * FROM users
WHERE city IS NOT NULL AND id IS NOT NULL
PRIMARY KEY (city, id);
-- Update the age of the user with id 1 and set a custom timestamp
UPDATE users USING TIMESTAMP 1234567890 SET age = 32 WHERE id = 1;
3. Neo4j Cypher Query Language
Overview and Features
Neo4j Cypher Query Language, simply known as Cypher, is a declarative graph query language designed specifically for Neo4j, a leading graph database management system. Cypher is designed to efficiently query, manipulate, and store graph data, which consists of nodes and relationships between them. Cypher's syntax is visually appealing and intuitive, allowing developers to focus on expressing the structure and patterns found within the data rather than writing complex queries.
Key features of Cypher include:
- A declarative and intuitive syntax for expressing graph patterns and relationships
- Support for pattern matching, filtering, and aggregation
- Built-in graph algorithms for efficient traversal and analytics
- Extensibility through user-defined procedures and functions
Basic Operations
Cypher supports a wide range of CRUD operations for managing data in a Neo4j graph database. Some common Cypher operations include:
MATCH
: Retrieve nodes and relationships from the graph that match a specified patternCREATE
: Create new nodes and relationships in the graphSET
: Update the properties of nodes or relationships that match a specified patternDELETE
: Delete nodes, relationships, or properties from the graph that match a specified pattern
Here's an example of how to use these basic Cypher operations:
-- Find all nodes with the label "User"
MATCH (u:User) RETURN u;
-- Create a new node with the label "User" and properties
CREATE (:User {name: 'Alice', age: 30, city: 'New York'});
-- Update the age of the user named "Alice"
MATCH (u:User {name: 'Alice'}) SET u.age = 31;
-- Delete the user node with the name "Alice"
MATCH (u:User {name: 'Alice'}) DETACH DELETE u;
Advanced Operations
In addition to basic CRUD operations, Cypher offers a rich set of advanced features and operations for complex graph analytics and traversal. Some examples of advanced Cypher operations include:
SHORTESTPATH
: Find the shortest path between two nodes based on a specified relationship type and criteriaALLSHORTESTPATHS
: Find all shortest paths between two nodes based on a specified relationship type and criteriaAPOC
procedures: Use the APOC (Awesome Procedures On Cypher) library to extend Cypher with custom procedures and functions
Here's an example demonstrating the use of advanced Cypher operations:
-- Find the shortest path between two users based on their friendship relationships
MATCH (a:User {name: 'Alice'}), (b:User {name: 'Bob'}),
path = shortestPath((a)-[:FRIEND*..5]-(b))
RETURN path;
-- Find all shortest paths between two users based on their friendship relationships
MATCH (a:User {name: 'Alice'}), (b:User {name: 'Bob'}),
paths = allShortestPaths((a)-[:FRIEND*..5]-(b))
RETURN paths;
-- Use an APOC procedure to find all users connected to a specific user within a specified degree of separation
MATCH (a:User {name: 'Alice'})
CALL apoc.path.subgraphAll(a, {relationshipFilter: 'FRIEND', minLevel: 1, maxLevel: 3})
YIELD nodes, relationships
RETURN nodes, relationships;
4. Couchbase N1QL (Non-First Normal Form Query Language)
Overview and Features
Couchbase N1QL (pronounced "nickel") is a powerful, SQL-like query language designed for Couchbase Server, a high-performance, distributed NoSQL document database. N1QL allows developers to query JSON-based documents using a familiar SQL-like syntax, making it easy to work with complex, nested, and heterogeneous data structures. Couchbase N1QL provides powerful indexing and query optimization capabilities, allowing for efficient and flexible data retrieval and manipulation.
Key features of Couchbase N1QL include:
- SQL-like syntax for querying and manipulating JSON-based documents
- Support for complex, nested, and heterogeneous data structures
- Advanced indexing capabilities, including Global Secondary Indexes (GSI)
- Extensibility through user-defined functions (UDFs) and integration with other data processing tools
Basic Operations
N1QL supports a wide range of CRUD operations for managing data in a Couchbase database. Some common N1QL operations include:
SELECT
: Retrieve documents from a bucket that match a specified filter or criteriaINSERT
: Insert a new document into a bucketUPDATE
: Update the values of fields in documents that match a specified filterDELETE
: Delete documents from a bucket that match a specified filter
Here's an example of how to use these basic N1QL operations:
-- Find all documents in the "users" bucket
SELECT * FROM users;
-- Insert a new document into the "users" bucket
INSERT INTO users (KEY, VALUE) VALUES ("user_alice", {"name": "Alice", "age": 30, "city": "New York"});
-- Update the age of the user named "Alice"
UPDATE users SET age = 31 WHERE name = "Alice";
-- Delete the document with the key "user_alice" from the "users" bucket
DELETE FROM users WHERE META().id = "user_alice";
Advanced Operations
Couchbase N1QL offers a rich set of advanced features and operations for working with complex, nested, and heterogeneous data structures. Some examples of advanced N1QL operations include:
NEST
: Combine documents from two buckets based on a specified join condition and create a nested JSON resultUNNEST
: Flatten a nested JSON array or object within a document into multiple rowsARRAY_AGG
: Aggregate values from multiple documents into a single JSON arrayOBJECT_PUT
: Add or replace a key-value pair in a JSON object
Here's an example demonstrating the use of advanced N1QL operations:
-- Find the total number of orders per user and their details, and sort by total in descending order
SELECT users.name, ARRAY_AGG(orders.orderId) AS orderIds, COUNT(orders.orderId) AS totalOrders, SUM(orders.amount) AS totalAmount
FROM users
NEST orders ON KEYS users.orderIds
GROUP BY users.name
ORDER BY totalAmount DESC;
-- Flatten the "interests" array within each user document into separate rows
SELECT u.name, interest
FROM users u
UNNEST u.interests AS interest;
-- Add a new field "country" with the value "USA" to all user documents
UPDATE users SET country = "USA";
5. Amazon DynamoDB Query Language
Overview and Features
Amazon DynamoDB is a fully managed, serverless NoSQL database service provided by Amazon Web Services (AWS). DynamoDB offers fast and consistent performance, automatic scaling, and is designed to handle large amounts of data and traffic. While DynamoDB does not have a dedicated query language like SQL, it provides a set of APIs and SDKs for various programming languages to perform CRUD operations and advanced data retrieval and manipulation tasks.
Key features of Amazon DynamoDB include:
- Fully managed and serverless NoSQL database with automatic scaling
- Support for key-value and document data models
- Consistent, low-latency performance for read and write operations
- Fine-grained access control and integration with other AWS services
Basic Operations
DynamoDB offers a comprehensive set of APIs and SDKs for performing CRUD operations on data stored in DynamoDB tables. Some common DynamoDB operations include:
GetItem
: Retrieve a single item from a table by its primary keyPutItem
: Create a new item or replace an existing item in a tableUpdateItem
: Update one or more attributes of an item in a tableDeleteItem
: Delete a single item from a table by its primary key
Here's an example of how to use these basic DynamoDB operations using the AWS SDK for JavaScript:
const AWS = require('aws-sdk');
const dynamoDB = new AWS.DynamoDB.DocumentClient();
// Get an item with a specific primary key from the "users" table
dynamoDB.get({
TableName: 'users',
Key: { id: 'user_alice' }
}, (err, data) => {
console.log(data.Item);
});
// Put a new item into the "users" table
dynamoDB.put({
TableName: 'users',
Item: { id: 'user_alice', name: 'Alice', age: 30, city: 'New York' }
}, (err, data) => {
console.log('Item inserted');
});
// Update the age of the user with the primary key "user_alice"
dynamoDB.update({
TableName: 'users',
Key: { id: 'user_alice' },
UpdateExpression: 'SET age = :new_age',
ExpressionAttributeValues: { ':new_age': 31 }
}, (err, data) => {
console.log('Item updated');
});
// Delete the item with the primary key "user_alice" from the "users" table
dynamoDB.delete({
TableName: 'users',
Key: { id: 'user_alice' }
}, (err, data) => {
console.log('Item deleted');
});
Advanced Operations
In addition to basic CRUD operations, DynamoDB provides APIs and SDKs for more advanced data retrieval and manipulation tasks, such as querying and scanning data based on various conditions and filters. Some examples of advanced DynamoDB operations include:
Query
: Retrieve items from a table using a specified primary key value or a range of primary key valuesScan
: Retrieve all items from a table, optionally filtering the results based on one or more conditionsFilterExpression
: Define conditions for filtering the results of a query or scan operation
Here's an example demonstrating the use of advanced DynamoDB operations:
// Retrieve all users with the city "New York"
dynamoDB.query({
TableName: 'users',
IndexName: 'city-index',
KeyConditionExpression: 'city = :city',
ExpressionAttributeValues: { ':city': 'New York' }
}, (err, data) => {
console.log(data.Items);
});
// Scan the "users" table and filter the results based on a specific age
dynamoDB.scan({
TableName: 'users',
FilterExpression: 'age >= :min_age',
ExpressionAttributeValues: { ':min_age': 30 }
}, (err, data) => {
console.log(data.Items);
});
Conclusion
In this article, we have explored five powerful NoSQL query languages for efficient data retrieval and manipulation:
- MongoDB Query Language (MQL)
- Cassandra Query Language (CQL)
- Neo4j Cypher Query Language
- Couchbase N1QL (Non-First Normal Form Query Language)
- Amazon DynamoDB Query Language
Each query language offers its unique set of features, capabilities, and syntax tailored to the specific NoSQL database it is designed for. When choosing a NoSQL query language for your project, it's essential to consider the data model, performance requirements, and the complexity of the data you will be working with.
By familiarizing yourself with these query languages and their features, you can better leverage the power of NoSQL databases and build more efficient, scalable, and flexible applications.
Frequently Asked Questions
1. What are the main differences between SQL and NoSQL query languages?
SQL query languages, such as SQL for relational databases, follow a structured and standardized syntax for querying and manipulating data. They rely on a fixed schema and relational model, making them ideal for handling structured data.
On the other hand, NoSQL query languages are designed for NoSQL databases, which do not follow a fixed schema or relational model. These languages have diverse syntax and functionality tailored to their respective NoSQL databases, such as document-based, key-value, column-family, or graph-based data models. NoSQL query languages provide flexibility in handling unstructured, semi-structured, and complex data structures.
2. Can I use a SQL query language with a NoSQL database?
Some NoSQL databases, such as Couchbase and Cassandra, offer SQL-like query languages (N1QL and CQL, respectively) that allow developers familiar with SQL to work with NoSQL databases more easily. However, these SQL-like query languages are tailored to their specific NoSQL databases and may have different syntax, features, and limitations compared to traditional SQL.
3. How do I choose the right NoSQL query language for my project?
When choosing a NoSQL query language for your project, consider the following factors:
- The type of NoSQL database you are using (document, key-value, column-family, or graph)
- The data model and structure of your data
- The complexity of the queries and data manipulation tasks you will perform
- The performance, scalability, and availability requirements of your application
By evaluating these factors, you can choose the appropriate NoSQL query language that best suits your project's needs.
4. Can I use multiple NoSQL query languages in a single project?
Yes, it is possible to use multiple NoSQL query languages in a single project, especially if you are using a polyglot persistence approach, where different databases are used for different data storage and processing needs. In such cases, you may need to use different query languages to interact with each NoSQL database. However, this approach may increase the complexity of your project and require a deeper understanding of each query language and its features.
5. How can I learn more about NoSQL query languages?
To learn more about NoSQL query languages, consider the following resources:
- Official documentation and tutorials for each NoSQL database and query language
- Online courses, webinars, and workshops on NoSQL databases and query languages
- Books, articles, and blog posts covering NoSQL database concepts, use cases, and best practices
- Community forums, discussion boards, and social media groups dedicated to NoSQL databases and query languages
By exploring these resources, you can deepen your understanding of NoSQL query languages and their applications in various domains.